帮我生成一个c++企业信息管理系统
时间: 2024-01-24 08:04:27 浏览: 21
好的,企业信息管理系统通常包含以下模块:员工信息管理、部门信息管理、考勤管理、薪资管理、招聘管理、培训管理等。下面是一个简单的C++企业信息管理系统的示例代码,你可以根据自己的需求进行修改和完善:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
//员工类
class Employee {
public:
Employee(string name, string gender, int age, string department) {
this->name = name;
this->gender = gender;
this->age = age;
this->department = department;
}
//获取员工姓名
string getName() const {
return name;
}
//获取员工性别
string getGender() const {
return gender;
}
//获取员工年龄
int getAge() const {
return age;
}
//获取员工所在部门
string getDepartment() const {
return department;
}
private:
string name;
string gender;
int age;
string department;
};
//员工信息管理类
class EmployeeManager {
public:
//添加员工
void addEmployee(Employee employee) {
employees.push_back(employee);
}
//删除员工
void deleteEmployee(string name) {
for (auto it = employees.begin(); it != employees.end(); it++) {
if (it->getName() == name) {
employees.erase(it);
break;
}
}
}
//查找员工
Employee* findEmployee(string name) {
for (auto it = employees.begin(); it != employees.end(); it++) {
if (it->getName() == name) {
return &(*it);
}
}
return nullptr;
}
//获取所有员工信息
vector<Employee> getAllEmployees() const {
return employees;
}
private:
vector<Employee> employees;
};
//部门类
class Department {
public:
Department(string name, string manager) {
this->name = name;
this->manager = manager;
}
//获取部门名称
string getName() const {
return name;
}
//获取部门经理
string getManager() const {
return manager;
}
private:
string name;
string manager;
};
//部门信息管理类
class DepartmentManager {
public:
//添加部门
void addDepartment(Department department) {
departments.push_back(department);
}
//删除部门
void deleteDepartment(string name) {
for (auto it = departments.begin(); it != departments.end(); it++) {
if (it->getName() == name) {
departments.erase(it);
break;
}
}
}
//查找部门
Department* findDepartment(string name) {
for (auto it = departments.begin(); it != departments.end(); it++) {
if (it->getName() == name) {
return &(*it);
}
}
return nullptr;
}
//获取所有部门信息
vector<Department> getAllDepartments() const {
return departments;
}
private:
vector<Department> departments;
};
int main() {
EmployeeManager employeeManager;
DepartmentManager departmentManager;
//添加员工和部门信息
employeeManager.addEmployee(Employee("张三", "男", 25, "研发部"));
employeeManager.addEmployee(Employee("李四", "女", 30, "市场部"));
departmentManager.addDepartment(Department("研发部", "王五"));
departmentManager.addDepartment(Department("市场部", "赵六"));
//输出所有员工信息
cout << "所有员工信息:" << endl;
for (auto employee : employeeManager.getAllEmployees()) {
cout << employee.getName() << " " << employee.getGender() << " " << employee.getAge() << " " << employee.getDepartment() << endl;
}
//输出所有部门信息
cout << "所有部门信息:" << endl;
for (auto department : departmentManager.getAllDepartments()) {
cout << department.getName() << " " << department.getManager() << endl;
}
//查找员工和部门信息
string name = "张三";
Employee* employee = employeeManager.findEmployee(name);
if (employee != nullptr) {
cout << "员工:" << name << " 性别:" << employee->getGender() << " 年龄:" << employee->getAge() << " 部门:" << employee->getDepartment() << endl;
} else {
cout << "未找到员工:" << name << endl;
}
name = "研发部";
Department* department = departmentManager.findDepartment(name);
if (department != nullptr) {
cout << "部门:" << name << " 经理:" << department->getManager() << endl;
} else {
cout << "未找到部门:" << name << endl;
}
//删除员工和部门信息
name = "张三";
employeeManager.deleteEmployee(name);
cout << "删除员工:" << name << endl;
name = "市场部";
departmentManager.deleteDepartment(name);
cout << "删除部门:" << name << endl;
//输出所有员工信息
cout << "所有员工信息:" << endl;
for (auto employee : employeeManager.getAllEmployees()) {
cout << employee.getName() << " " << employee.getGender() << " " << employee.getAge() << " " << employee.getDepartment() << endl;
}
//输出所有部门信息
cout << "所有部门信息:" << endl;
for (auto department : departmentManager.getAllDepartments()) {
cout << department.getName() << " " << department.getManager() << endl;
}
return 0;
}
```
以上是一个简单的C++企业信息管理系统示例代码,你可以根据自己的需求进行修改和完善。
相关推荐
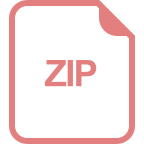
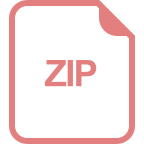














