Python实训小结
时间: 2024-07-02 16:01:17 浏览: 176
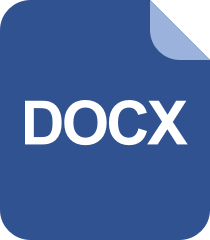
Python实训周总结.docx
在Python实训中,通常会涵盖以下几个核心内容的小结:
1. **基础语法**:学习Python的基础结构,包括变量、数据类型(如整数、浮点数、字符串、列表、字典等)、控制流(if-else、for循环、while循环)、函数定义和调用。
2. **模块和标准库**:理解如何导入和使用内置模块,如math、os、sys等,以及第三方库如numpy、pandas和matplotlib等,用于数据分析和图形化。
3. **面向对象编程**:介绍类和对象的概念,封装、继承、多态性,以及如何创建和使用类的方法和属性。
4. **异常处理**:学习如何使用try-except-finally语句捕获和处理程序运行中的错误。
5. **文件操作**:处理文件读写,包括打开、关闭、追加、读取、写入等操作。
6. **网络编程**:了解socket编程,如果涉及,可能还会学习HTTP或WebSockets通信。
7. **数据结构和算法**:掌握常用的数据结构,如队列、栈、链表、树和图,以及简单的算法实现。
8. **项目实战**:通过实际项目,如爬虫、数据分析、Web应用开发等,应用所学知识,提升实践能力。
阅读全文
相关推荐
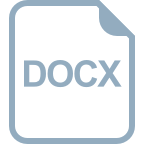
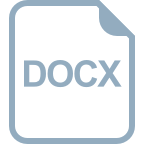
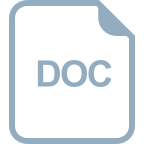
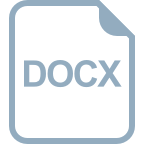
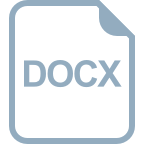
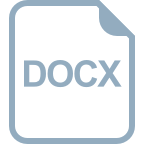
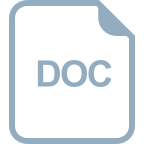
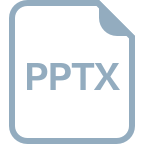
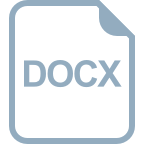
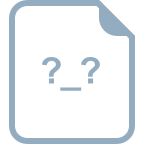


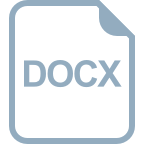
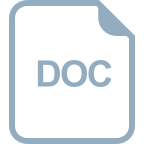
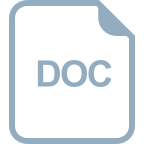
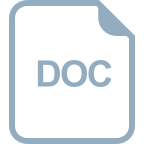
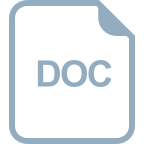
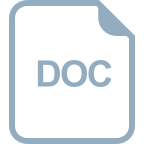
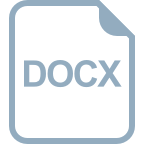