dev-c++写流星雨代码
时间: 2023-12-01 18:37:58 浏览: 68
Sure, I can help you write code for a meteor shower. Here's a simple example in C:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define NUM_METEORS 10
typedef struct {
int x;
int y;
} Meteor;
void generateMeteors(Meteor* meteors) {
srand(time(NULL));
for (int i = 0; i < NUM_METEORS; i++) {
meteors[i].x = rand() % 100;
meteors[i].y = rand() % 100;
}
}
void drawMeteors(Meteor* meteors) {
printf("Meteor shower:\n");
for (int i = 0; i < NUM_METEORS; i++) {
printf("Meteor %d: Position (%d, %d)\n", i+1, meteors[i].x, meteors[i].y);
}
}
int main() {
Meteor meteors[NUM_METEORS];
generateMeteors(meteors);
drawMeteors(meteors);
return 0;
}
```
This code defines a struct `Meteor` to represent a meteor with coordinates `x` and `y`. The `generateMeteors` function randomly generates the positions of the meteors, and the `drawMeteors` function prints out the positions. Finally, in the `main` function, we create an array of meteors, generate their positions, and then draw them.
Related questions:
1. How can I modify the code to increase or decrease the number of meteors?
2. Is there a way to animate the meteor shower on the screen?
3. How can I calculate the speed and direction of each meteor?
相关推荐
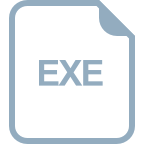
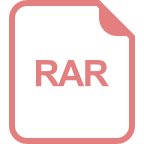














