Java中如何使用序列化技术实现深克隆,给出示例代码
时间: 2024-11-23 18:38:34 浏览: 27
在Java中,序列化通常用于将对象转换为字节流以便存储或传输,而深克隆则是创建一个全新的、与原对象完全独立的对象副本。虽然Java的默认序列化机制并不能直接实现深克隆,但是可以借助第三方库如`java.beans.XMLDecoder`或者`ObjectInputStream`和`ObjectOutputStream`配合,通过反序列化的方式达到类似深拷贝的效果。
下面是一个简单的示例,使用`ObjectOutputStream`和`ObjectInputStream`实现深克隆:
```java
import java.io.*;
public class DeepCloneExample {
private String value;
// ... 省略getter和setter
public static Object deepClone(Object obj) throws IOException, ClassNotFoundException {
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
ObjectOutputStream objectOutputStream = new ObjectOutputStream(byteArrayOutputStream);
objectOutputStream.writeObject(obj);
byte[] serializedBytes = byteArrayOutputStream.toByteArray();
ByteArrayInputStream byteArrayInputStream = new ByteArrayInputStream(serializedBytes);
ObjectInputStream objectInputStream = new ObjectInputStream(byteArrayInputStream);
return objectInputStream.readObject();
}
public static void main(String[] args) {
try {
DeepCloneExample original = new DeepCloneExample();
original.value = "Hello, World!";
DeepCloneExample cloned = (DeepCloneExample) deepClone(original);
System.out.println("Original: " + original.value); // 输出 "Hello, World!"
System.out.println("Cloned: " + cloned.value); // 输出 "Hello, World!" (新实例,值相同)
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
```
在这个例子中,我们首先序列化原始对象,然后从序列化的字节数组中反序列化得到一个新的对象,实现了深复制。注意,这个方法只适用于实现了Serializable接口的类,而且这种方法并不是所有复杂的对象结构都能完美复制,对于一些不可序列化的属性,或者需要特殊处理的类,还需要额外的代码来处理。
阅读全文
相关推荐
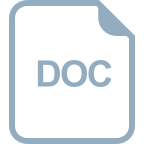
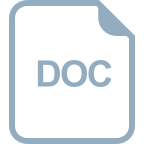
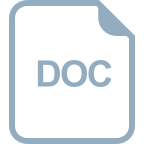
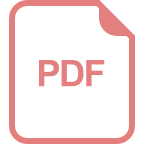
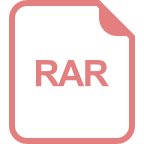
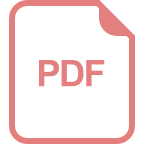
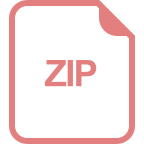
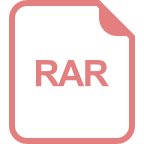
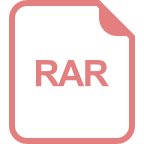
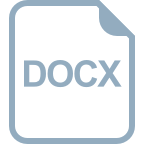
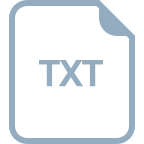
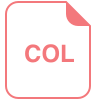
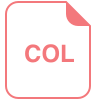
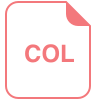
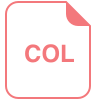
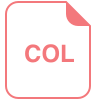
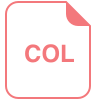

