用三个c++程序分别说明异步通信中三种不同的互锁方式
时间: 2024-09-29 10:13:36 浏览: 39
在C++中,异步通信通常涉及线程间的协作,为了保证数据的一致性和资源安全,需要对并发操作进行适当的同步控制。以下是三种常见的互斥方式,每个示例会展示一个简单的场景:
1. **互斥量(Mutex)**:
```cpp
#include <mutex>
#include <thread>
std::mutex mtx; // 创建一个互斥量
void print_num(int num) {
std::lock_guard<std::mutex> lock(mtx); // 获取锁
std::cout << "Thread " << std::this_thread::get_id() << ": " << num << std::endl;
}
int main() {
std::thread t1(print_num, 10);
std::thread t2(print_num, 20);
t1.join(); // 等待t1完成
t2.join(); // 等待t2完成
return 0;
}
```
在这个例子中,`mtx`确保了两个线程不会同时访问打印数字的代码。
2. **条件变量(Condition Variable)**:
```cpp
#include <condition_variable>
#include <queue>
std::queue<int> numbers;
std::mutex mtx;
std::condition_variable cv;
void producer(int value) {
std::unique_lock<std::mutex> lock(mtx);
// 生产者填满队列
for (int i = 0; i < value; ++i)
numbers.push(i);
lock.unlock();
cv.notify_all(); // 唤醒所有等待者
}
void consumer() {
std::unique_lock<std::mutex> lock(mtx);
while (numbers.empty()) { // 消费者阻塞直到有元素
cv.wait(lock);
}
int num = numbers.front();
lock.unlock();
std::cout << "Thread " << std::this_thread::get_id() << ": Consumed " << num << std::endl;
}
int main() {
std::thread producer_thread(producer, 5);
std::thread consumer_thread(consumer);
producer_thread.join();
consumer_thread.join();
return 0;
}
```
这里,条件变量允许生产者在填满队列后唤醒消费者。
3. **信号量(Semaphore)**:
```cpp
#include <semaphore>
#include <thread>
std::semaphore sema(1); // 初始化一个信号量值为1
void shared_resource_access() {
sema.acquire(); // 获得信号量
std::cout << "Thread " << std::this_thread::get_id() << " acquired" << std::endl;
sema.release(); // 释放信号量
}
int main() {
std::thread t1(shared_resource_access);
std::thread t2(shared_resource_access);
t1.join();
t2.join();
return 0;
}
```
信号量通过获取和释放的方式来限制同时访问共享资源的线程数。
阅读全文
相关推荐
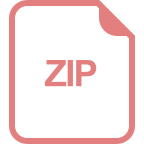
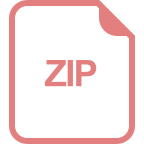

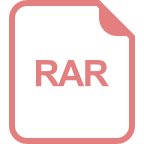
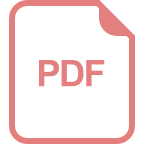
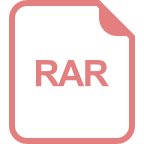
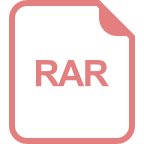
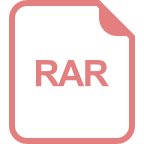
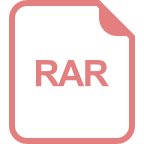
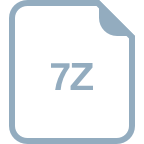
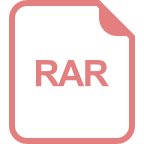
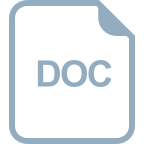
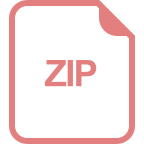
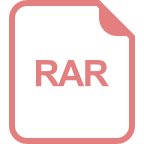
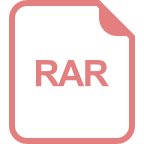
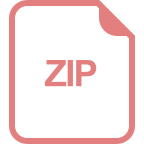