单片机使用sram原理和代码
时间: 2024-04-10 13:34:02 浏览: 30
单片机使用SRAM(Static Random Access Memory)是一种静态随机存取存储器,它可以用来存储数据。下面是使用SRAM的原理和代码示例:
原理:
1. SRAM是一种易失性存储器,它使用闪存电容来存储数据。
2. 单片机通过地址总线将要读取或写入的内存地址发送给SRAM。
3. 单片机通过数据总线将数据发送给SRAM进行写操作,或者从SRAM读取数据。
代码示例(以C语言为例):
```c
#include <avr/io.h> // AVR单片机的IO头文件
#define SRAM_ADDRESS 0x2000 // SRAM的起始地址
// 初始化SRAM
void init_sram() {
// 设置地址总线和数据总线的方向为输出
DDRA = 0xFF; // 地址总线为8位
DDRB = 0xFF; // 数据总线为8位
}
// 从SRAM读取数据
unsigned char read_sram(unsigned int address) {
// 设置地址总线的值
PORTA = (address >> 8) & 0xFF; // 高8位
PORTB = address & 0xFF; // 低8位
// 读取SRAM数据
return PINB;
}
// 向SRAM写入数据
void write_sram(unsigned int address, unsigned char data) {
// 设置地址总线的值
PORTA = (address >> 8) & 0xFF; // 高8位
PORTB = address & 0xFF; // 低8位
// 写入SRAM数据
PORTB = data;
}
int main() {
init_sram();
unsigned int address = SRAM_ADDRESS;
unsigned char data = 0x55;
// 向SRAM写入数据
write_sram(address, data);
// 从SRAM读取数据
unsigned char readData = read_sram(address);
// 输出读取到的数据
printf("Read data: 0x%x\n", readData);
return 0;
}
```
以上是使用SRAM的简单原理和代码示例,注意具体的实现可能会因单片机型号和编译器而有所不同。请根据实际情况进行适当调整。
相关推荐
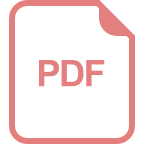
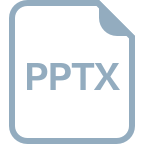
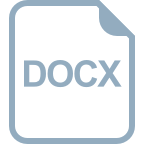
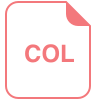













