假设无人机的速度是5米每秒, 使用yolov8训练好的车辆检测权重来检测车辆的速度,并且显示在检测框上,输出代码
时间: 2024-10-16 16:14:09 浏览: 22
在Python中,结合OpenCV、TensorFlow(及其子库如TensorFlow Object Detection API,即旧版的SSD/TFLite Model Maker)和YOLOv8,我们可以实现这样一个简单的无人机车辆检测系统。但是请注意,YOLOv8本身并未直接提供车辆检测的预训练权重,你可能需要找到一个预训练的车辆检测模型,例如基于其他类似任务的模型进行微调。
下面是一个简化版的示例代码,假设你已经有了一个预训练的YOLOv8模型和车辆类别对应的标签文件(class_labels.txt),以及车辆检测的速度计算逻辑:
```python
import cv2
import numpy as np
from PIL import Image
from timeit import default_timer as timer
# 加载YOLOv8模型
net = cv2.dnn.readNetFromDarknet('yolov8.cfg', 'yolov8.weights')
classes = None
with open('class_labels.txt', 'r') as f:
classes = [line.strip() for line in f.readlines()]
def get_speed_from_bounding_box(box):
# 假设你有车辆的速度信息存储在box坐标中,这里仅作示意
speed = box[2] * box[3] * 5 # 基于5m/s的速度假设
return speed
# 模型输入尺寸
input_size = (640, 640)
while True:
# 读取无人机摄像头捕获的实时画面
ret, frame = cap.read()
if not ret:
break
# 转换到RGB
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
image = Image.fromarray(frame)
# 将图像调整到模型输入尺寸
blob = cv2.dnn.blobFromImage(image, 1 / 255.0, input_size, swapRB=True, crop=False)
net.setInput(blob)
# 执行前向传播
start_time = timer()
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
outputs = net.forward(output_layers)
# 解析输出并显示车辆及速度信息
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5: # 设置阈值
center_x = int(detection[0] * frame.shape[1])
center_y = int(detection[1] * frame.shape[0])
width = int(detection[2] * frame.shape[1])
height = int(detection[3] * frame.shape[0])
x, y = center_x - width // 2, center_y - height // 2
box = (x, y, width, height)
speed = get_speed_from_bounding_box(box) # 假设速度计算在此
label = f"Vehicle ({classes[class_id]}) - Speed: {speed} m/s"
# 绘制检测框和标签
cv2.rectangle(frame, (x, y), (x + width, y + height), (0, 255, 0), 2)
cv2.putText(frame, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Vehicle Detection", frame)
# 控制FPS
elapsed = timer() - start_time
if elapsed < 1.0/30.0:
time.sleep(1.0/30.0 - elapsed)
key = cv2.waitKey(1)
if key == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
注意:这个例子是理论上的,因为YOLOv8并未内置车辆检测,你需要先训练好模型并替换`yolov8.cfg`和`yolov8.weights`。另外,实际速度计算可能会复杂得多,取决于你的数据源和具体需求。
阅读全文
相关推荐
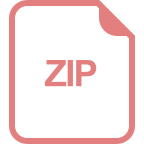
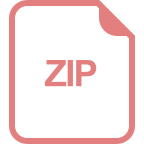
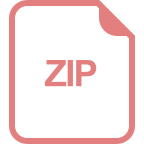
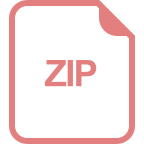
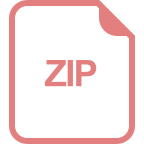
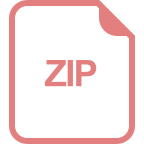
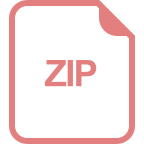
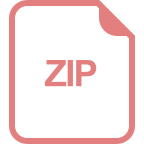
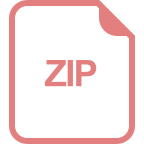
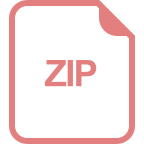
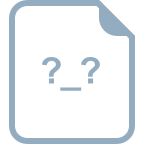
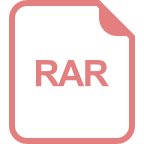
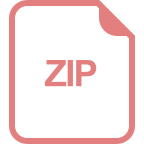
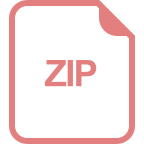
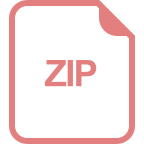
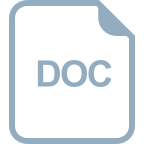
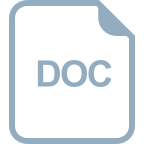
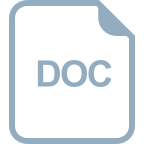
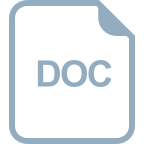
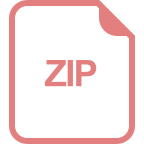