c语言修改文件中的某个字符串
时间: 2023-12-12 22:05:42 浏览: 176
以下是使用C语言修改文件中某个字符串的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
FILE *fp;
char buffer[BUFFER_SIZE];
char *tempName = "temp.txt";
char *str1 = argv[1]; // 需要替换的字符串
char *str2 = argv[2]; // 替换成的字符串
// 打开原始文件
fp = fopen(argv[3], "r");
if (fp == NULL) {
printf("Failed to open file: %s\n", argv[3]);
exit(1);
}
// 创建临时文件
FILE *temp = fopen(tempName, "w");
if (temp == NULL) {
printf("Failed to create temporary file.\n");
fclose(fp);
exit(1);
}
// 逐行读取原始文件并替换字符串
while (fgets(buffer, BUFFER_SIZE, fp)) {
char *pos = strstr(buffer, str1);
while (pos != NULL) {
int index = pos - buffer;
memmove(pos + strlen(str2), pos + strlen(str1), strlen(pos) - strlen(str1) + 1);
memcpy(pos, str2, strlen(str2));
pos = strstr(pos + strlen(str2), str1);
}
fputs(buffer, temp);
}
// 关闭文件
fclose(fp);
fclose(temp);
// 删除原始文件并重命名临时文件
remove(argv[3]);
rename(tempName, argv[3]);
printf("String replaced successfully.\n");
return 0;
}
```
上述代码中,我们使用了`fgets()`函数逐行读取原始文件,并使用`strstr()`函数查找需要替换的字符串。如果找到了需要替换的字符串,我们使用`memmove()`函数将后面的字符向后移动,然后使用`memcpy()`函数将新的字符串复制到原始字符串的位置。最后,我们将修改后的内容写入临时文件中,并在完成替换后删除原始文件并重命名临时文件。
阅读全文
相关推荐
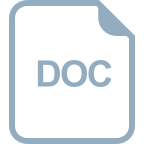
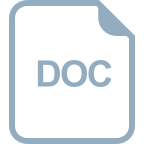
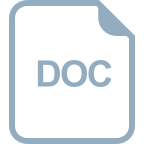

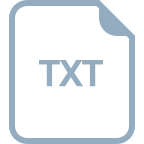
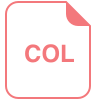
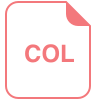


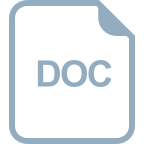
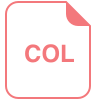
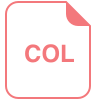
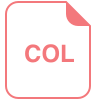
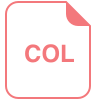
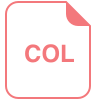
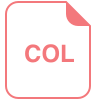
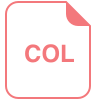
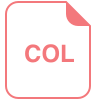
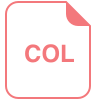