vue怎么用<image>设置背景图片
时间: 2024-11-25 16:31:42 浏览: 4
在Vue.js中,如果你想使用`<image>`标签来设置背景图片,实际上这不是Vue官方推荐的方式,因为`<image>`通常是用于显示网络图像的。然而,如果你确实需要将其用作背景,可以结合CSS来实现。首先,创建一个`<div>`或者其他容器元素,然后将`<image>`作为其内容,并为其设置CSS背景属性。
HTML部分示例:
```html
<div class="background-image">
<image :src="imageUrl" slot="image-source"></image>
</div>
```
在这里,`:src`是一个指令,它会动态绑定到`imageUrl`的数据属性。
CSS部分:
```css
.background-image {
background-image: url({{ imageUrl }}); /* 使用双花括号语法插入动态路径 */
background-size: cover; /* 如果你想让图片铺满容器 */
background-repeat: no-repeat;
}
```
注意,由于Vue不直接支持HTML5的`<image>`标签,所以最好在实际项目中考虑使用更常见的`<img>`标签配合样式或者`v-bind:class`来改变背景。
相关问题
改正代码<template> <div style="background-image: ".image/image1.jpg""> </div> </template> <script setup></script> <style></style>
这段代码看起来像是 Vue.js 的模板部分,但是存在一些语法错误。`<template>` 标签用于定义 HTML 元素和组件的结构,而 `style` 属性在 Vue 中应该使用 `v-bind` 或者 `:` 来绑定 CSS 属性。下面是修复后的代码:
```html
<template>
<div :style="{ 'background-image': `url(${image1})` }">
<!-- 在这里添加其他内容,如果需要 -->
</div>
</template>
<script setup>
import { ref } from 'vue';
// 如果 image1 是一个变量
const image1 = ref("image1.jpg");
// 如果是导入一个对象,例如
// const images = { image1: "image1.jpg" };
// 可以这样引用
// const image1 = images.image1;
</script>
<style scoped>
/* 如果需要编写内联样式,可以在这里 */
</style>
```
在这个修复版本中,我做了以下更改:
1. 将 `style` 属性改为 `:style`,并使用对象字面量设置背景图片的 URL,使用 Vue 的插值表达式 `${}` 和变量 `image1`。
2. 使用 `ref` 或其他数据管理方法引入需要的数据,如图片路径。
3. 添加了 `scoped` 到 `<style>` 标签上,防止样式污染全局。
我是程序员,帮我在vue3环境下添加一下显示控件,播放的时候能下方显示声音,播放键,全屏<template> <div> <video ref="videoPlayer" @ended="playNextVideo"> <source :src="currentVideoSrc" type="video/mp4"> </video> </div> </template> <script> export default { data() { return { currentVideoIndex: 0, // 当前播放的视频索引 videoList: [ // 视频列表 { src: '/src/video/666.mp4' }, { src: '/src/video/777.mp4' }, { src: '' } ], autoplayInterval: null // 自动播放的定时器 } }, computed: { currentVideoSrc() { return this.videoList[this.currentVideoIndex].src } }, mounted() { this.$refs.videoPlayer.play() // 自动播放 this.autoplayInterval = setInterval(() => { this.playNextVideo() }, 5000) }, beforeUnmount() { // 清除定时器 clearInterval(this.autoplayInterval) }, methods: { playNextVideo() { this.currentVideoIndex++ if (this.currentVideoIndex >= this.videoList.length) { this.currentVideoIndex = 0 } this.$refs.videoPlayer.src = this.currentVideoSrc this.$refs.videoPlayer.play() } } } </script>
好的,你已经有一个简单的视频播放器的代码了,接下来我会指导你如何在 Vue3 环境下添加显示控件,包括声音、播放键和全屏。
首先,你需要引入一个支持控件的第三方库,例如 video.js 或者 Plyr。然后,你可以按照以下步骤添加控件:
1. 在你的 Vue 组件中,添加一个 `div` 元素,用于包裹视频播放器和控件。
2. 在该 `div` 元素中,添加控件元素,例如声音控件、播放键控件和全屏控件。
3. 在控件元素中,使用第三方库提供的 API,设置控件的相关属性,例如样式、位置等。
以下是一个示例代码:
```html
<template>
<div class="player-wrapper">
<div class="player-controls">
<button class="player-button player-volume"></button>
<button class="player-button player-play"></button>
<button class="player-button player-fullscreen"></button>
</div>
<video ref="videoPlayer" @ended="playNextVideo">
<source :src="currentVideoSrc" type="video/mp4">
</video>
</div>
</template>
<script>
import videojs from 'video.js';
import 'video.js/dist/video-js.css';
export default {
data() {
return {
currentVideoIndex: 0,
videoList: [
{
src: '/src/video/666.mp4'
},
{
src: '/src/video/777.mp4'
},
{
src: ''
}
],
autoplayInterval: null
}
},
computed: {
currentVideoSrc() {
return this.videoList[this.currentVideoIndex].src
}
},
mounted() {
this.player = videojs(this.$refs.videoPlayer, {
controls: false
});
this.$refs.videoPlayer.play();
this.autoplayInterval = setInterval(() => {
this.playNextVideo()
}, 5000);
},
beforeUnmount() {
clearInterval(this.autoplayInterval)
},
methods: {
playNextVideo() {
this.currentVideoIndex++;
if (this.currentVideoIndex >= this.videoList.length) {
this.currentVideoIndex = 0;
}
this.player.src({
src: this.currentVideoSrc,
type: 'video/mp4'
});
this.player.play();
}
}
};
</script>
<style>
.player-wrapper {
position: relative;
width: 100%;
height: 0;
padding-top: 56.25%; /* 16:9 aspect ratio */
}
.player-controls {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
height: 50px;
display: flex;
justify-content: space-between;
align-items: center;
background-color: rgba(0, 0, 0, 0.5);
padding: 0 20px;
box-sizing: border-box;
}
.player-button {
width: 30px;
height: 30px;
border: none;
background-color: transparent;
background-size: contain;
background-repeat: no-repeat;
cursor: pointer;
}
.player-volume {
background-image: url('/src/images/volume.png');
}
.player-play {
background-image: url('/src/images/play.png');
}
.player-fullscreen {
background-image: url('/src/images/fullscreen.png');
}
.video-js {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
</style>
```
在上述代码中,我们使用了 video.js 库来实现播放器功能。我们将视频播放器和控件都放在了一个包裹元素中,并设置其样式,以使其按照 16:9 的比例显示,并且控件显示在视频播放器的下方。
我们使用 `controls: false` 选项来关闭 video.js 默认的控件显示,并且在播放器的 `mounted` 钩子中,初始化一个播放器实例。在 `methods` 中,我们使用 `this.player` 来访问播放器实例,并设置 `src` 属性来播放视频。我们在 `playNextVideo` 方法中,按照顺序播放视频列表中的视频,并且在视频播放结束时自动播放下一个视频。
在样式中,我们设置了控件元素的样式和位置,并且使用背景图片来显示控件图标。你可以根据自己的需要调整控件的样式、位置等。
希望这能够帮助你实现在 Vue3 环境下添加一个带有控件的视频播放器。
阅读全文
相关推荐
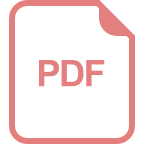
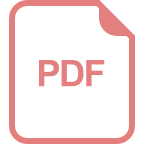
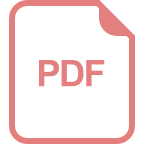













