C++不适用锁实现建立三个线程A、B、C,A线程打印10次字母A,B线程打印10次字母B,C线程打印10次字母C,但是要求三个线程同时运行,并且实现交替打印,即按照ABCABCABC的顺序打印。
时间: 2024-03-04 13:48:15 浏览: 99
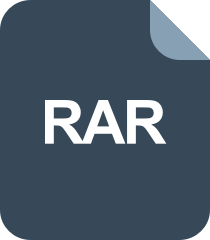
文件打印问题 (c++多线程实现)

这里提供一种基于原子变量和条件变量的解法,避免了使用锁。
```cpp
#include <iostream>
#include <thread>
#include <atomic>
#include <condition_variable>
std::atomic<int> count(0);
const int kMaxCount = 30;
std::condition_variable cv;
std::mutex cv_mtx;
void print(char ch, int num) {
for (int i = 0; i < num; ++i) {
while (count % 3 != ch - 'A') {
std::unique_lock<std::mutex> lock(cv_mtx);
cv.wait(lock);
}
std::cout << ch;
++count;
cv.notify_all();
}
}
int main() {
std::thread threadA(print, 'A', 10);
std::thread threadB(print, 'B', 10);
std::thread threadC(print, 'C', 10);
threadA.join();
threadB.join();
threadC.join();
std::cout << std::endl;
return 0;
}
```
在这个解法中,我们使用了一个`std::atomic<int>`类型的变量`count`来记录当前应该打印哪个字符。同时,我们使用了一个`std::condition_variable`类型的变量`cv`和一个`std::mutex`类型的变量`cv_mtx`来实现线程的等待和唤醒。
在`print`函数内部,我们使用了一个`while`循环来判断是否轮到当前线程打印字符。如果不是,就等待唤醒。如果是,就打印字符并递增`count`,然后通过`cv.notify_all()`来唤醒其他线程。
在`main`函数中,我们创建三个线程并分别传入不同的字符和打印次数,然后等待三个线程执行完毕后输出换行符。如果一切正常,程序应该输出:`ABCABCABCABCABCABCABCABCABCABC`。
注意,这种解法使用了原子变量和条件变量,可以避免使用锁。但是,它可能会带来额外的开销和复杂性,具体取决于系统的实现。在实际应用中,需要根据具体情况进行选择。
阅读全文
相关推荐
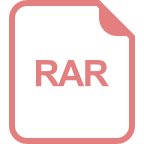
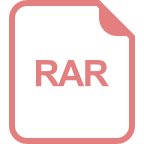


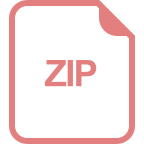



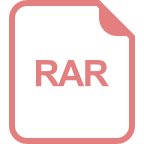
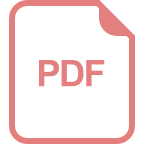
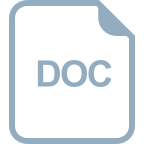





