springboot基于GraphHopper实现地理编码代码
时间: 2023-07-11 13:23:30 浏览: 172
GraphHopper是一个开源的路线规划引擎,也可以用来进行地理编码。下面介绍如何在SpringBoot中基于GraphHopper实现地理编码。
首先需要在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.graphhopper</groupId>
<artifactId>graphhopper-core</artifactId>
<version>3.0.0</version>
</dependency>
```
然后在SpringBoot的配置文件中,需要添加以下配置项:
```yml
graphhopper:
graph:
location: /path/to/graphhopper-data
```
这里的`/path/to/graphhopper-data`是GraphHopper的数据文件存放路径,可以使用GraphHopper提供的命令行工具来生成数据文件。
接着,在Service类中,可以使用GraphHopper的API来实现地理编码:
```java
import com.graphhopper.GHResponse;
import com.graphhopper.GraphHopper;
import com.graphhopper.PathWrapper;
import com.graphhopper.reader.osm.GraphHopperOSM;
import com.graphhopper.routing.util.EncodingManager;
import com.graphhopper.util.PointList;
import com.graphhopper.util.shapes.GHPoint;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
@Service
public class GeoCoderService {
@Value("${graphhopper.graph.location}")
private String graphHopperLocation;
private GraphHopper graphHopper;
@Autowired
public GeoCoderService(GraphHopper graphHopper) {
this.graphHopper = graphHopper;
}
public GHPoint geocode(double lat, double lng) {
graphHopper.load(graphHopperLocation);
GHResponse response = graphHopper.route(
new GHRequest(lat, lng)
.setAlgorithm("astar")
.setVehicle("car")
.setWeighting("fastest")
.setLocale(Locale.US)
);
PointList pointList = response.getBest().getPoints();
return new GHPoint(pointList.getLat(0), pointList.getLon(0));
}
}
```
在上面的代码中,使用GraphHopper的API来进行地理编码。在构造`GHRequest`对象时,需要传入经纬度和路线规划算法、交通工具、权重等参数。在返回结果中,使用`getBest()`方法获取最优路径,并使用`getPoints()`方法获取路径的起点坐标。
最后,在Controller中可以使用`GeoCoderService`来进行地理编码:
```java
@RestController
@RequestMapping("/api")
public class GeoCoderController {
@Autowired
private GeoCoderService geoCoderService;
@GetMapping("/geocode")
public GHPoint geocode(@RequestParam("lat") double lat, @RequestParam("lng") double lng) {
return geoCoderService.geocode(lat, lng);
}
}
```
在上面的代码中,通过传入经纬度来进行地理编码。最终返回的是路径的起点坐标。
阅读全文
相关推荐
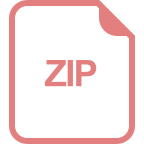
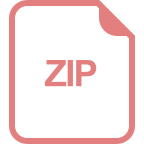
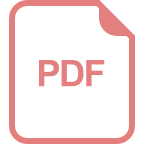















