c++多态和单例结合
时间: 2024-01-03 20:04:44 浏览: 135
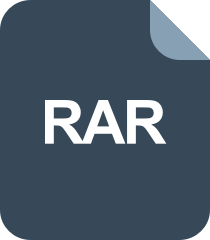
c++ Design-Patterns.rar
C++中的多态和单例模式可以结合使用,具体实现方式如下:
1. 定义一个抽象基类,其中包含一个静态的指向该类的唯一实例的指针。
2. 在该抽象基类中定义一个静态的获取唯一实例的方法,该方法返回该类的唯一实例。
3. 在派生类中重写该方法,返回该派生类的唯一实例。
4. 在使用时,通过抽象基类的静态方法获取唯一实例,然后调用该实例的虚函数,实现多态。
示例代码如下:
```c++
class Singleton {
public:
static Singleton* getInstance() {
if (instance == nullptr) {
instance = new Singleton();
}
return instance;
}
virtual void doSomething() = 0;
protected:
Singleton() {}
static Singleton* instance;
};
Singleton* Singleton::instance = nullptr;
class Derived : public Singleton {
public:
static Derived* getInstance() {
if (instance == nullptr) {
instance = new Derived();
}
return dynamic_cast<Derived*>(instance);
}
void doSomething() override {
std::cout << "Derived do something" << std::endl;
}
private:
Derived() {}
};
int main() {
Singleton* s = Derived::getInstance();
s->doSomething();
return 0;
}
```
阅读全文
相关推荐
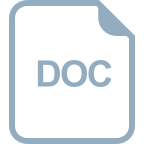
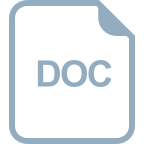
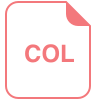
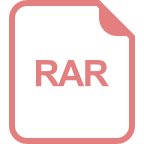
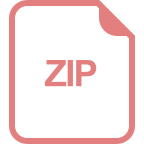
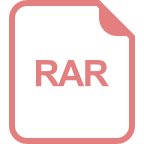
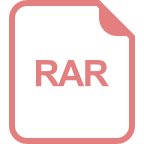
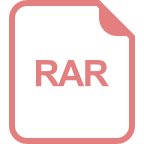
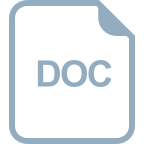
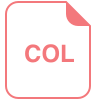
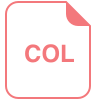
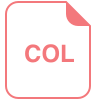
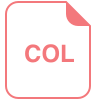
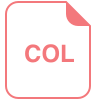
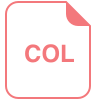
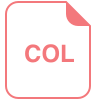
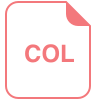
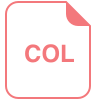