具体学生一卡通管理系统
时间: 2023-07-10 08:33:02 浏览: 78
好的,下面我为您提供一份基于C++的学生一卡通管理系统的代码示例,希望能对您有所帮助。
```c++
#include <iostream>
#include <vector>
#include <string>
#include <ctime>
using namespace std;
// 学生类
class Student {
private:
string name; // 姓名
int id; // 学号
string department; // 学院
public:
Student(string name, int id, string department) {
this->name = name;
this->id = id;
this->department = department;
}
string getName() {
return name;
}
int getId() {
return id;
}
string getDepartment() {
return department;
}
void setName(string name) {
this->name = name;
}
void setId(int id) {
this->id = id;
}
void setDepartment(string department) {
this->department = department;
}
};
// 一卡通类
class Card {
private:
int id; // 卡号
int balance; // 余额
bool isLost; // 是否挂失
vector<Record> records; // 消费记录
public:
Card(int id, int balance) {
this->id = id;
this->balance = balance;
this->isLost = false;
}
int getId() {
return id;
}
int getBalance() {
return balance;
}
bool getIsLost() {
return isLost;
}
void setId(int id) {
this->id = id;
}
void setBalance(int balance) {
this->balance = balance;
}
void setIsLost(bool isLost) {
this->isLost = isLost;
}
void recharge(int amount) {
balance += amount;
}
void consume(int amount) {
if (!isLost && balance >= amount) {
balance -= amount;
time_t now = time(0);
tm *ltm = localtime(&now);
string time = to_string(ltm->tm_year + 1900) + "-" + to_string(ltm->tm_mon + 1) + "-" + to_string(ltm->tm_mday) + " " + to_string(ltm->tm_hour) + ":" + to_string(ltm->tm_min) + ":" + to_string(ltm->tm_sec);
Record record(id, amount, time);
records.push_back(record);
}
}
vector<Record> getRecords() {
return records;
}
};
// 消费记录类
class Record {
private:
int cardId; // 卡号
int amount; // 消费金额
string time; // 消费时间
public:
Record(int cardId, int amount, string time) {
this->cardId = cardId;
this->amount = amount;
this->time = time;
}
int getCardId() {
return cardId;
}
int getAmount() {
return amount;
}
string getTime() {
return time;
}
};
// 学生一卡通管理系统类
class StudentCardSystem {
private:
vector<Student> students; // 学生信息
vector<Card> cards; // 一卡通信息
public:
void addStudent(Student student) {
students.push_back(student);
}
void addCard(Card card) {
cards.push_back(card);
}
Student getStudentById(int id) {
for (int i = 0; i < students.size(); i++) {
if (students[i].getId() == id) {
return students[i];
}
}
Student student("", 0, "");
return student;
}
Card getCardById(int id) {
for (int i = 0; i < cards.size(); i++) {
if (cards[i].getId() == id) {
return cards[i];
}
}
Card card(0, 0);
return card;
}
void recharge(int cardId, int amount) {
Card card = getCardById(cardId);
card.recharge(amount);
for (int i = 0; i < cards.size(); i++) {
if (cards[i].getId() == cardId) {
cards[i] = card;
}
}
}
void consume(int cardId, int amount) {
Card card = getCardById(cardId);
card.consume(amount);
for (int i = 0; i < cards.size(); i++) {
if (cards[i].getId() == cardId) {
cards[i] = card;
}
}
}
vector<Record> getRecords(int cardId) {
Card card = getCardById(cardId);
return card.getRecords();
}
};
// 主函数
int main() {
// 初始化学生一卡通管理系统
StudentCardSystem system;
Student student1("张三", 1001, "计算机科学与技术");
Student student2("李四", 1002, "软件工程");
system.addStudent(student1);
system.addStudent(student2);
Card card1(10001, 100);
Card card2(10002, 200);
system.addCard(card1);
system.addCard(card2);
// 充值
system.recharge(10001, 50);
system.recharge(10002, 100);
// 消费
system.consume(10001, 30);
system.consume(10002, 50);
// 查询消费记录
vector<Record> records1 = system.getRecords(10001);
vector<Record> records2 = system.getRecords(10002);
cout << "消费记录:" << endl;
cout << "卡号\t消费金额\t消费时间" << endl;
for (int i = 0; i < records1.size(); i++) {
cout << records1[i].getCardId() << "\t" << records1[i].getAmount() << "\t" << records1[i].getTime() << endl;
}
for (int i = 0; i < records2.size(); i++) {
cout << records2[i].getCardId() << "\t" << records2[i].getAmount() << "\t" << records2[i].getTime() << endl;
}
return 0;
}
```
以上代码仅供参考,具体实现还需根据实际需求进行调整。
阅读全文
相关推荐
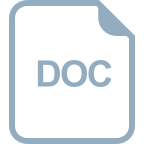
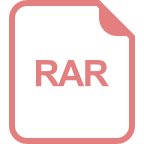
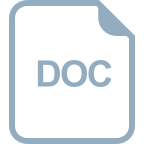





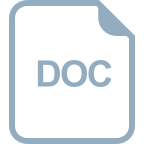
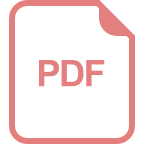
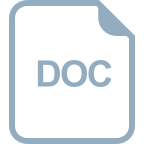
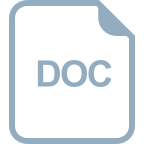
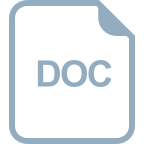
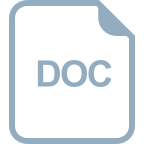