用python设计一个带有纹理贴图的六面体,在空中一边自传一边沿椐圆轨道绕地球运行的过程
时间: 2024-03-14 14:47:56 浏览: 43
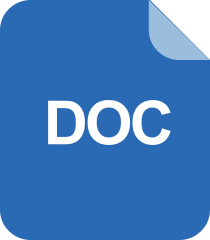
正六面体贴图openGL
要实现这个需求,需要使用 Python 的三维图形库,比如 `PyOpenGL` 和 `PyGame`,以及一些数学库,比如 `numpy`。
以下是一个简单的实现过程:
1. 导入必要的库
```python
import numpy as np
import pygame
from pygame.locals import *
from OpenGL.GL import *
from OpenGL.GLU import *
```
2. 初始化 Pygame 和 OpenGL
```python
pygame.init()
display = (800, 600)
pygame.display.set_mode(display, DOUBLEBUF | OPENGL)
gluPerspective(45, (display[0] / display[1]), 0.1, 50.0)
glTranslatef(0.0, 0.0, -5)
```
3. 定义六面体的坐标和纹理
```python
vertices = np.array([
[-1, -1, -1],
[1, -1, -1],
[1, 1, -1],
[-1, 1, -1],
[-1, -1, 1],
[1, -1, 1],
[1, 1, 1],
[-1, 1, 1]
], dtype=np.float32)
texcoords = np.array([
[0, 0],
[1, 0],
[1, 1],
[0, 1]
], dtype=np.float32)
faces = np.array([
[0, 1, 2, 3],
[3, 2, 6, 7],
[7, 6, 5, 4],
[4, 5, 1, 0],
[1, 5, 6, 2],
[4, 0, 3, 7]
], dtype=np.int32)
```
4. 加载纹理并启用纹理映射
```python
texture_surface = pygame.image.load('texture.png')
texture_data = pygame.image.tostring(texture_surface, 'RGBA', 1)
width = texture_surface.get_width()
height = texture_surface.get_height()
texture_id = glGenTextures(1)
glBindTexture(GL_TEXTURE_2D, texture_id)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, width, height, 0, GL_RGBA, GL_UNSIGNED_BYTE, texture_data)
glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE)
glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE)
glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glEnable(GL_TEXTURE_2D)
```
5. 定义自转和公转的初始状态
```python
self_rotation = 0
revolution = 0
revolution_speed = 0.1
```
6. 绘制六面体并添加自转和公转
```python
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
glEnable(GL_DEPTH_TEST)
glRotatef(self_rotation, 1, 1, 1)
glTranslatef(0, 0, 4)
glRotatef(revolution, 0, 1, 0)
glTranslatef(0, 0, -4)
glBegin(GL_QUADS)
for face in faces:
for i, vertex in enumerate(face):
glTexCoord2fv(texcoords[i])
glVertex3fv(vertices[vertex])
glEnd()
pygame.display.flip()
self_rotation += 1
revolution += revolution_speed
pygame.time.wait(10)
```
7. 运行程序并观察六面体在空中自传一边沿椐圆轨道绕地球运行的过程
这个实现过程只是一个简单的例子,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
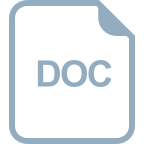
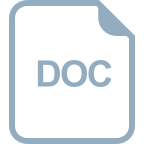




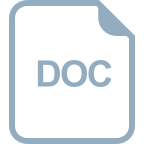
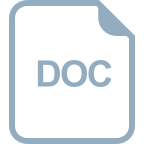
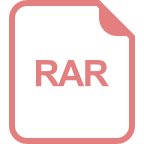
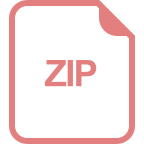