用C语言实现AES加密
时间: 2024-05-13 13:18:45 浏览: 94
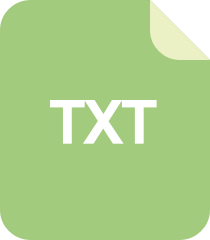
AES加密C语言实现
实现AES加密需要用到AES算法库。以下是一个使用OpenSSL库的C语言程序,实现对数据进行AES加密:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
int main(int argc, char *argv[]) {
// 待加密的数据
unsigned char input[] = "Hello, world!";
// 密钥
unsigned char key[] = "0123456789abcdef";
// 向量
unsigned char iv[] = "fedcba9876543210";
// 初始化AES算法上下文
AES_KEY aes_key;
if (AES_set_encrypt_key(key, 128, &aes_key) < 0) {
fprintf(stderr, "Unable to set encryption key in AES\n");
exit(EXIT_FAILURE);
}
// 加密数据
unsigned char *output = malloc(strlen(input) + 1);
memset(output, 0, strlen(input) + 1);
AES_cbc_encrypt(input, output, strlen(input), &aes_key, iv, AES_ENCRYPT);
// 输出加密后的结果
printf("Input: %s\n", input);
printf("Output: ");
for (int i = 0; i < strlen(input); i++) {
printf("%02x", output[i]);
}
printf("\n");
free(output);
return 0;
}
```
在运行程序之前,需要先安装OpenSSL库。在Linux系统中,可以通过以下命令安装:
```
sudo apt-get install libssl-dev
```
编译程序:
```
gcc -o aes aes.c -lcrypto
```
然后运行程序:
```
./aes
```
程序输出的结果将会是一个十六进制的字符串,即为加密后的数据。
阅读全文
相关推荐


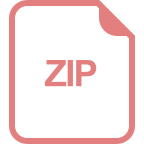


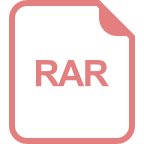
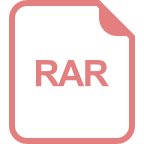
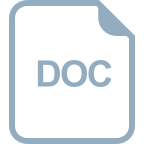
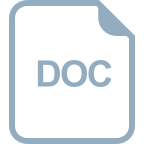
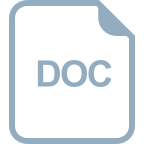
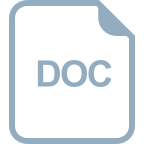

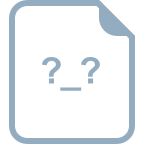
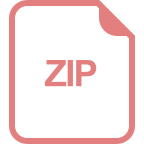