vue2弹窗并实现分页
时间: 2023-07-02 20:19:59 浏览: 158
你可以使用第三方的弹窗组件库,例如 Element UI 或者 Vuetify。这些组件库都提供了弹窗组件,并且可以轻松地在 Vue 中使用。
对于分页的实现,你可以使用 Vue 的计算属性来计算当前页要显示的数据。根据你的具体需求,你可以在弹窗中嵌入一个分页组件,或者手动编写分页逻辑。
以下是一个示例代码,使用 Element UI 的弹窗组件和分页组件:
```html
<template>
<div>
<el-button @click="showDialog">打开弹窗</el-button>
<el-dialog :visible.sync="dialogVisible">
<el-table :data="pagedData" border>
<el-table-column prop="id" label="ID"></el-table-column>
<el-table-column prop="name" label="名称"></el-table-column>
</el-table>
<el-pagination
:current-page.sync="currentPage"
:page-size="pageSize"
:total="total"
@current-change="handleCurrentChange"
></el-pagination>
</el-dialog>
</div>
</template>
<script>
import { reactive, computed } from 'vue';
import { Dialog, Table, TableColumn, Pagination, Button } from 'element-ui';
import axios from 'axios';
export default {
components: {
ElDialog: Dialog,
ElTable: Table,
ElTableColumn: TableColumn,
ElPagination: Pagination,
ElButton: Button,
},
setup() {
const state = reactive({
dialogVisible: false,
data: [], // 所有数据
currentPage: 1, // 当前页码
pageSize: 10, // 每页数量
});
// 获取数据
const fetchData = async () => {
const res = await axios.get('/api/data');
state.data = res.data;
};
// 计算当前页要显示的数据
const pagedData = computed(() => {
const start = (state.currentPage - 1) * state.pageSize;
const end = start + state.pageSize;
return state.data.slice(start, end);
});
// 数据总数
const total = computed(() => state.data.length);
// 处理当前页码变化
const handleCurrentChange = (page) => {
state.currentPage = page;
};
// 打开弹窗
const showDialog = () => {
fetchData();
state.dialogVisible = true;
};
return {
dialogVisible: state.dialogVisible,
pagedData,
currentPage: state.currentPage,
pageSize: state.pageSize,
total,
handleCurrentChange,
showDialog,
};
},
};
</script>
```
在这个示例中,我们使用了 Element UI 的弹窗组件和分页组件,以及 Axios 库来获取数据。在 `setup` 函数中,我们使用了 Vue 3 的新特性 `reactive` 来创建响应式对象,以及 `computed` 来计算当前页要显示的数据和数据总数。在模板中,我们嵌入了一个表格和一个分页组件,在弹窗中显示数据。当用户点击分页组件的页码时,我们会更新当前页码,同时计算出新的要显示的数据。
阅读全文
相关推荐
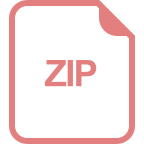
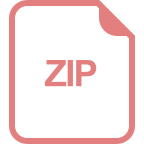
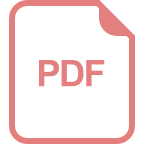
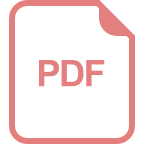
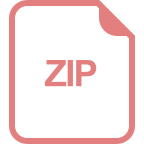
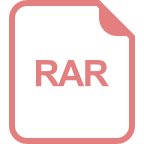
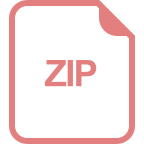
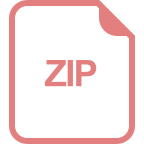
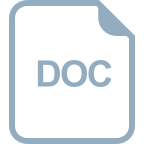
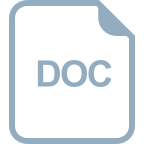
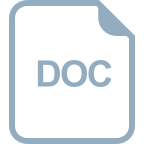
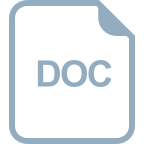
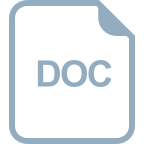
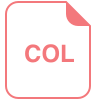
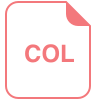
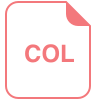