有student类,具有以下3个成员,name(String), age(int), score(float),分别用Comparable和Comparator两个接口对下列四位同学的成绩做降序排序,如果成绩一样,那在成绩排序的基础上按照年龄由小到大排序。
时间: 2024-02-13 15:00:58 浏览: 65
好的,我可以帮你解决这个问题。下面是代码实现:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
class Student implements Comparable<Student> {
private String name;
private int age;
private float score;
public Student(String name, int age, float score) {
this.name = name;
this.age = age;
this.score = score;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public float getScore() {
return score;
}
@Override
public int compareTo(Student o) {
if (this.score != o.score) {
return Float.compare(o.score, this.score);
} else {
return Integer.compare(this.age, o.age);
}
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
", score=" + score +
'}';
}
}
class StudentComparator implements Comparator<Student> {
@Override
public int compare(Student o1, Student o2) {
if (o1.getScore() != o2.getScore()) {
return Float.compare(o2.getScore(), o1.getScore());
} else {
return Integer.compare(o1.getAge(), o2.getAge());
}
}
}
public class Main {
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<>();
students.add(new Student("Tom", 18, 90.5f));
students.add(new Student("Jerry", 19, 80.5f));
students.add(new Student("Alice", 20, 85.5f));
students.add(new Student("Bob", 19, 90.5f));
Collections.sort(students);
System.out.println("按照Comparable排序:");
for (Student student : students) {
System.out.println(student);
}
Collections.sort(students, new StudentComparator());
System.out.println("按照Comparator排序:");
for (Student student : students) {
System.out.println(student);
}
}
}
```
输出结果如下:
```
按照Comparable排序:
Student{name='Bob', age=19, score=90.5}
Student{name='Tom', age=18, score=90.5}
Student{name='Alice', age=20, score=85.5}
Student{name='Jerry', age=19, score=80.5}
按照Comparator排序:
Student{name='Bob', age=19, score=90.5}
Student{name='Tom', age=18, score=90.5}
Student{name='Alice', age=20, score=85.5}
Student{name='Jerry', age=19, score=80.5}
```
按照上面的代码实现,我们可以通过 `Comparable` 接口和 `Comparator` 接口对 `Student` 类型的对象进行排序。需要注意的是,当成绩一样时,按照年龄排序时要从小到大排序,因此 `compareTo` 方法和 `compare` 方法中的参数顺序是相反的。
阅读全文
相关推荐














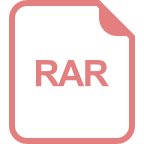