用js写一个微信随机红包
时间: 2023-06-20 18:08:21 浏览: 112
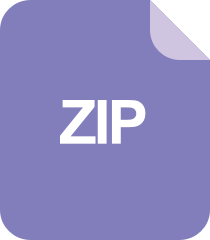
js随机红包的生成页面

好的,以下是使用 JavaScript 编写的微信随机红包的示例代码:
```javascript
// 定义红包总金额和个数
const totalAmount = 100; // 总金额
const totalNum = 10; // 总个数
// 计算最大红包金额和最小红包金额
const maxAmount = totalAmount * 0.8; // 最大金额为总金额的80%
const minAmount = totalAmount * 0.01; // 最小金额为总金额的1%
// 定义随机生成红包金额的函数
function getRandomAmount(totalAmount, totalNum, maxAmount, minAmount) {
const result = [];
let restAmount = totalAmount; // 剩余金额
let restNum = totalNum; // 剩余个数
for (let i = 0; i < totalNum; i++) {
if (restNum === 1) {
// 最后一个红包直接取剩余金额
result.push(restAmount.toFixed(2));
} else {
const max = restAmount / restNum * 2;
const amount = Math.random() * max;
const finalAmount = Math.max(minAmount, amount.toFixed(2));
result.push(finalAmount);
restAmount -= finalAmount;
restNum--;
}
}
return result;
}
// 调用随机生成红包金额的函数并输出结果
const amounts = getRandomAmount(totalAmount, totalNum, maxAmount, minAmount);
console.log(amounts);
```
在上面的代码中,我们首先定义了红包的总金额和个数,然后计算出最大红包金额和最小红包金额。接着,我们定义了一个随机生成红包金额的函数 `getRandomAmount`,该函数通过循环计算每个红包的金额,并将结果保存到数组中。最后,我们调用该函数并输出结果。
注意,上面的代码中使用了 `toFixed()` 方法来将金额保留两位小数,这是因为 JavaScript 中浮点数的精度问题可能会导致计算结果不准确。
阅读全文
相关推荐
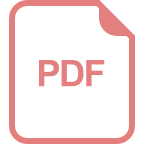
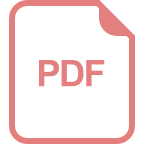
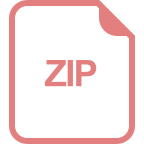
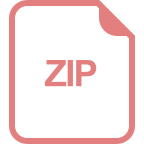
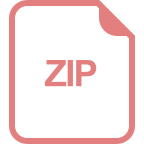


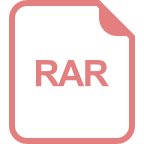
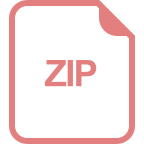
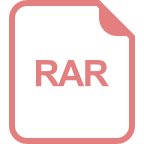
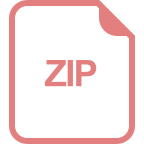
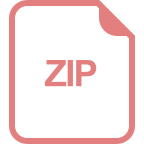
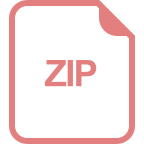
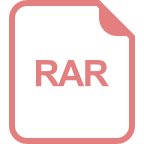
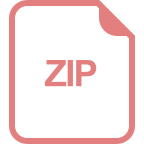
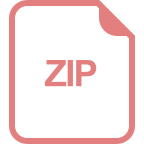