python的插值函数
时间: 2023-08-07 18:57:20 浏览: 54
Python中常用的插值函数有多种,其中一种常见的是使用scipy库中的插值函数。scipy库提供了许多不同类型的插值方法,包括线性插值、多项式插值、样条插值等。
以下是一个简单的示例,展示如何使用scipy库进行线性插值:
```python
import numpy as np
from scipy.interpolate import interp1d
# 定义原始数据
x = np.array([1, 2, 3, 4, 5])
y = np.array([2, 4, 1, 5, 3])
# 创建插值函数
f = interp1d(x, y)
# 在新的x值上进行插值
x_new = np.linspace(1, 5, num=50)
y_new = f(x_new)
# 输出插值结果
print(y_new)
```
在这个例子中,我们首先定义了一组原始数据 `x` 和 `y`,然后使用 `interp1d` 函数创建了一个插值函数 `f`。接下来,我们通过将新的 `x` 值传递给插值函数 `f` 来获取相应的 `y` 值,从而进行插值。最后,我们打印出了插值结果 `y_new`。
除了线性插值外,scipy库还提供了其他类型的插值方法,例如使用 `interp1d` 函数的 `kind` 参数可以指定为 `'cubic'` 来进行三次样条插值。
希望这个例子能帮助你理解如何在Python中使用插值函数。
相关问题
python插值函数
Python中常用的插值函数有很多种,比如线性插值、多项式插值、样条插值等。其中,scipy库中的interpolate模块提供了丰富的插值函数。
以线性插值为例,可以使用interp1d函数进行插值。示例代码如下:
```python
from scipy.interpolate import interp1d
x = [1, 2, 3, 4, 5]
y = [2, 4, 1, 3, 7]
f = interp1d(x, y)
print(f(2.5)) # 输出:2.5
```
以上代码中,x和y分别表示已知的数据点的横纵坐标,interp1d函数返回一个插值函数f,可以通过f(x)来计算x处的插值结果。
python插值函数代码
以下是Python中的插值函数代码示例:
1. 线性插值
```python
from scipy.interpolate import interp1d
# 输入数据
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 40, 50]
# 创建插值函数对象
f = interp1d(x, y)
# 计算插值结果
x_new = [1.5, 2.5, 3.5, 4.5]
y_new = f(x_new)
print(y_new)
```
2. 二次样条插值
```python
from scipy.interpolate import interp1d
from scipy.interpolate import interp1d
# 输入数据
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 40, 50]
# 创建插值函数对象
f = interp1d(x, y, kind='quadratic')
# 计算插值结果
x_new = [1.5, 2.5, 3.5, 4.5]
y_new = f(x_new)
print(y_new)
```
3. 三次样条插值
```python
from scipy.interpolate import interp1d
from scipy.interpolate import interp1d
# 输入数据
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 40, 50]
# 创建插值函数对象
f = interp1d(x, y, kind='cubic')
# 计算插值结果
x_new = [1.5, 2.5, 3.5, 4.5]
y_new = f(x_new)
print(y_new)
```
以上代码使用了SciPy库中的interp1d函数,可以实现线性插值、二次样条插值和三次样条插值。其中,kind参数可以设置为linear、quadratic和cubic,分别对应线性插值、二次样条插值和三次样条插值。
相关推荐
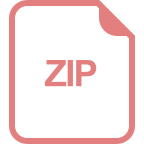
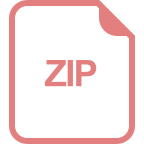
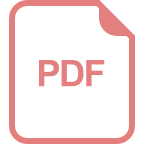












