Java可视化界面 把数据库信息打印在界面上、
时间: 2023-11-11 19:04:54 浏览: 62
要在Java可视化界面中打印数据库信息,需要使用Java Swing或JavaFX等GUI库进行界面设计,以及Java JDBC API进行数据库连接和操作。
以下是一个简单的例子,演示如何将数据库中的信息打印到Java Swing界面上:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
public class DatabaseTable extends JFrame {
private JTable table;
private JScrollPane scrollPane;
public DatabaseTable() {
setTitle("Database Table");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(500, 500);
try {
// Connect to the database
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
Statement stmt = conn.createStatement();
// Execute a query and get the result set
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable");
// Create a table model and fill it with the data from the result set
MyTableModel model = new MyTableModel(rs);
// Create a JTable with the table model
table = new JTable(model);
// Add the JTable to a JScrollPane
scrollPane = new JScrollPane(table);
// Add the JScrollPane to the JFrame
getContentPane().add(scrollPane);
// Close the database connection
rs.close();
stmt.close();
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
DatabaseTable frame = new DatabaseTable();
frame.setVisible(true);
}
}
class MyTableModel extends ResultSetTableModel {
public MyTableModel(ResultSet rs) {
super(rs);
}
@Override
public int getColumnCount() {
// Return the number of columns in the result set
try {
return getResultSet().getMetaData().getColumnCount();
} catch (Exception e) {
e.printStackTrace();
return 0;
}
}
@Override
public String getColumnName(int column) {
// Return the name of the specified column in the result set
try {
return getResultSet().getMetaData().getColumnName(column + 1);
} catch (Exception e) {
e.printStackTrace();
return "";
}
}
@Override
public Object getValueAt(int row, int column) {
// Return the value at the specified row and column in the result set
try {
getResultSet().absolute(row + 1);
return getResultSet().getObject(column + 1);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
```
在这个例子中,我们使用了MySQL数据库作为示例。你需要将代码中的数据库连接URL、用户名和密码替换为你自己的,以及将SELECT查询语句中的表名替换为你自己的表名。
这个例子中的MyTableModel类扩展了ResultSetTableModel类,该类是一个抽象类,提供了一个通用的实现,允许将任何ResultSet对象转换为TableModel对象。在MyTableModel类中,我们重写了getColumnCount()、getColumnName()和getValueAt()方法,以从结果集中获取列数、列名和单元格值。这些方法可根据你的需求进行修改。
最后,在main()方法中创建DatabaseTable对象并将其设置为可见。当你运行这个程序时,将显示一个包含数据库信息的表格。
相关推荐
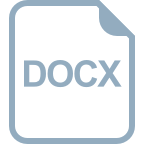
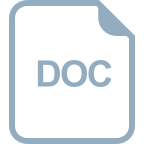
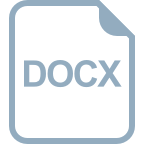














