threadLocal demo
时间: 2023-07-23 19:54:30 浏览: 109
ThreadLocal 是 Java 中的一个类,用于实现线程局部变量。它提供了一种在多线程环境下,每个线程都可以独立拥有自己的变量副本的机制。这意味着每个线程都可以访问和修改自己的变量副本,而不会影响其他线程的副本。
下面是一个简单的 ThreadLocal 的示例代码:
```java
public class ThreadLocalDemo {
private static ThreadLocal<Integer> threadLocal = new ThreadLocal<>();
public static void main(String[] args) {
// 在主线程中设置变量值
threadLocal.set(10);
// 创建两个新的线程,并分别获取和修改变量值
Thread thread1 = new Thread(() -> {
int value = threadLocal.get();
System.out.println("Thread 1: " + value); // 输出:Thread 1: 10
threadLocal.set(20);
System.out.println("Thread 1 updated value: " + threadLocal.get()); // 输出:Thread 1 updated value: 20
});
Thread thread2 = new Thread(() -> {
int value = threadLocal.get();
System.out.println("Thread 2: " + value); // 输出:Thread 2: null
threadLocal.set(30);
System.out.println("Thread 2 updated value: " + threadLocal.get()); // 输出:Thread 2 updated value: 30
});
thread1.start();
thread2.start();
// 在主线程中获取变量值
int value = threadLocal.get();
System.out.println("Main Thread: " + value); // 输出:Main Thread: 10
}
}
```
在上面的示例中,我们创建了一个 ThreadLocal 对象 `threadLocal`,并在主线程中设置了变量值为 10。然后我们创建了两个新的线程 `thread1` 和 `thread2`,它们分别获取和修改变量值。
注意到在 `thread2` 中,由于没有调用 `threadLocal.set()` 方法设置变量值,所以在获取变量值时返回的是 `null`。
最后,在主线程中再次获取变量值,可以看到它保持了初始设置的值 10。这说明每个线程都独立地拥有自己的变量副本。
希望这个示例能够帮助你理解 ThreadLocal 的使用方式和作用。如果还有其他问题,请继续提问。
阅读全文
相关推荐

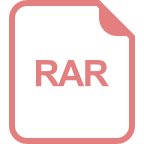
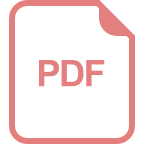
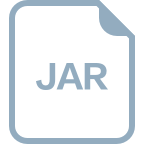
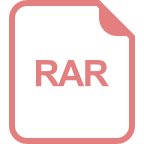
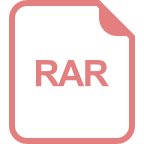
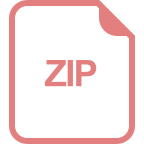
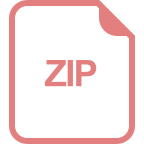
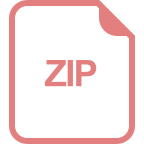
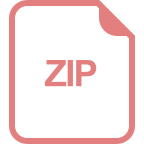
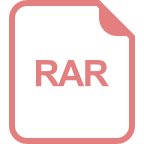
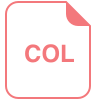



