android使用kotin实现完整的登录注册功能
时间: 2023-12-21 09:06:28 浏览: 174
要实现完整的登录注册功能,需要涉及到以下几个步骤:
1. 创建一个Android项目并添加Kotlin支持。
2. 设计用户界面。可以使用XML来定义布局和控件。
3. 创建一个数据模型类来存储用户信息。
```kotlin
data class User(val email: String, val password: String)
```
4. 创建一个数据库类来管理用户信息的存储和检索。
```kotlin
class UserDatabase(context: Context) : SQLiteOpenHelper(context, DATABASE_NAME, null, DATABASE_VERSION) {
companion object {
private const val DATABASE_VERSION = 1
private const val DATABASE_NAME = "UserDatabase"
private const val TABLE_NAME = "users"
private const val COLUMN_ID = "id"
private const val COLUMN_EMAIL = "email"
private const val COLUMN_PASSWORD = "password"
}
override fun onCreate(db: SQLiteDatabase?) {
val CREATE_USERS_TABLE = ("CREATE TABLE $TABLE_NAME ($COLUMN_ID INTEGER PRIMARY KEY AUTOINCREMENT, $COLUMN_EMAIL TEXT, $COLUMN_PASSWORD TEXT)")
db?.execSQL(CREATE_USERS_TABLE)
}
override fun onUpgrade(db: SQLiteDatabase?, oldVersion: Int, newVersion: Int) {
db?.execSQL("DROP TABLE IF EXISTS $TABLE_NAME")
onCreate(db)
}
fun addUser(user: User) {
val db = this.writableDatabase
val values = ContentValues()
values.put(COLUMN_EMAIL, user.email)
values.put(COLUMN_PASSWORD, user.password)
db.insert(TABLE_NAME, null, values)
db.close()
}
fun getUser(email: String): User? {
val db = this.readableDatabase
val cursor = db.query(TABLE_NAME, arrayOf(COLUMN_ID, COLUMN_EMAIL, COLUMN_PASSWORD), "$COLUMN_EMAIL=?", arrayOf(email), null, null, null, null)
return if (cursor != null && cursor.moveToFirst()) {
val id = cursor.getInt(cursor.getColumnIndex(COLUMN_ID))
val password = cursor.getString(cursor.getColumnIndex(COLUMN_PASSWORD))
User(email, password)
} else {
null
}
}
}
```
5. 创建一个注册活动和一个登录活动来处理用户的注册和登录请求。
```kotlin
class RegisterActivity : AppCompatActivity() {
private lateinit var database: UserDatabase
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_register)
database = UserDatabase(this)
btn_register.setOnClickListener {
val email = et_email.text.toString()
val password = et_password.text.toString()
val user = User(email, password)
database.addUser(user)
Toast.makeText(this, "Registered successfully", Toast.LENGTH_SHORT).show()
finish()
}
}
}
class LoginActivity : AppCompatActivity() {
private lateinit var database: UserDatabase
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_login)
database = UserDatabase(this)
btn_login.setOnClickListener {
val email = et_email.text.toString()
val password = et_password.text.toString()
val user = database.getUser(email)
if (user != null && user.password == password) {
Toast.makeText(this, "Logged in successfully", Toast.LENGTH_SHORT).show()
finish()
} else {
Toast.makeText(this, "Invalid email or password", Toast.LENGTH_SHORT).show()
}
}
}
}
```
6. 在AndroidManifest.xml文件中注册这两个活动。
```xml
<activity android:name=".RegisterActivity" />
<activity android:name=".LoginActivity" />
```
7. 在主界面上添加按钮来启动注册和登录活动。
```xml
<Button
android:id="@+id/btn_register"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Register"
android:onClick="register" />
<Button
android:id="@+id/btn_login"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Login"
android:onClick="login" />
```
8. 在MainActivity.kt文件中实现这两个按钮的点击事件。
```kotlin
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
fun register(view: View) {
val intent = Intent(this, RegisterActivity::class.java)
startActivity(intent)
}
fun login(view: View) {
val intent = Intent(this, LoginActivity::class.java)
startActivity(intent)
}
}
```
这样就完成了一个使用Kotlin实现的完整的登录注册功能。
阅读全文
相关推荐





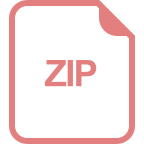
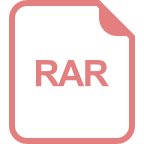
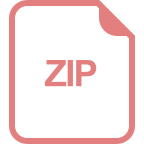







