#include <stdio.h> #include <stdlib.h> #define MAX_SIZE 100 // 线性表的最大长度 // 线性表结构体 typedef struct { int data[MAX_SIZE]; // 存储数据的数组 int length; // 线性表的长度 } List; // 初始化线性表 void initList(List *list) { list->length = 0; // 将线性表的长度初始化为0 } // 插入数据到线性表中 void insertList(List *list, int data) { if (list->length >= MAX_SIZE) { // 判断线性表是否已满 printf("List is full, cannot insert data.\n"); return; } list->data[list->length] = data; // 将数据插入到线性表中 list->length++; // 线性表的长度加1 } int main() { int n; // 数据的数量 List list; // 线性表 initList(&list); // 初始化线性表 printf("Please input the number of data: "); scanf("%d", &n); // 输入数据的数量 printf("Please input the data: "); for (int i = 0; i < n; i++) { int data; scanf("%d", &data); // 输入数据 insertList(&list, data); // 将数据插入到线性表中 } printf("The list is: "); for (int i = 0; i < list.length; i++) { printf("%d ", list.data[i]); // 输出线性表中的数据 } printf("\n"); return 0; } 以上代码中的List list; 是什么意思
时间: 2024-05-04 16:18:26 浏览: 97
List list; 表示声明了一个名为list的变量,其类型为List结构体。List结构体中包含一个存储数据的数组data和一个记录线性表长度的变量length。因此,List list; 的含义是声明了一个线性表变量list,可以通过该变量来操作线性表。在主函数中,通过调用initList函数初始化了该线性表,并且通过insertList函数向线性表中插入了一些数据。最后,通过遍历线性表的方式输出了线性表中的数据。
相关问题
#include <stdio.h> #include <stdlib.h> # include <malloc.h> #define MAXSIZE 100 #define OK 1 #define ERROR 0 #define TRUE 1 #define FALSE 0 typedef int ElemType; typedef int Status; typedef struct { ElemType *pList; int sqSize; int sqLength; }SqList; Status InitList(SqList *L); Status CreateList(SqList *L); Status PrintList(SqList *L); Status InsertList(SqList *L,int i,ElemType e); Status DeleteSqList(SqList *L,int i,ElemType *e); //初始化一个空的线性表 Status InitList(SqList *L) { L->pList = (SqList *)malloc(sizeof(SqList)); //申请内存空间 if(L->pList == NULL) { printf("内存空间分配失败!"); } else { L->sqLength = 0; } return OK; } 请分析这段代码哪里有误
这段代码有几个问题:
1. 在结构体定义中,`pList` 应该是 `ElemType` 类型的指针,而不是 `SqList` 类型的指针;
2. 在 `InitList` 函数中,应该先将 `sqSize` 赋值为 `MAXSIZE`,再分配内存空间;
3. 在 `InitList` 函数中,应该将 `pList` 赋值为一个 `ElemType` 类型的数组,而不是一个 `SqList` 类型的指针;
4. 在 `InitList` 函数中,应该将 `sqLength` 赋值为 0,而不是在 `else` 语句块中赋值;
5. 在 `InsertList` 函数中,应该判断插入位置 `i` 是否合法,即 `i > 0 && i <= L->sqLength + 1`;
6. 在 `InsertList` 函数中,应该判断线性表是否已满,即 `L->sqLength >= MAXSIZE`;
7. 在 `DeleteSqList` 函数中,应该判断删除位置 `i` 是否合法,即 `i > 0 && i <= L->sqLength`。
修正后的代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#define MAXSIZE 100
#define OK 1
#define ERROR 0
#define TRUE 1
#define FALSE 0
typedef int ElemType;
typedef int Status;
typedef struct {
ElemType *pList;
int sqSize;
int sqLength;
} SqList;
// 初始化一个空的线性表
Status InitList(SqList *L) {
L->pList = (ElemType *)malloc(sizeof(ElemType) * MAXSIZE); //申请内存空间
if (L->pList == NULL) {
printf("内存空间分配失败!");
return ERROR;
} else {
L->sqSize = MAXSIZE;
L->sqLength = 0;
return OK;
}
}
// 创建线性表
Status CreateList(SqList *L) {
printf("请输入线性表的长度:");
scanf("%d", &(L->sqLength));
printf("请输入线性表的元素:");
for (int i = 0; i < L->sqLength; i++) {
scanf("%d", &(L->pList[i]));
}
return OK;
}
// 输出线性表
Status PrintList(SqList *L) {
printf("线性表中的元素为:");
for (int i = 0; i < L->sqLength; i++) {
printf("%d ", L->pList[i]);
}
printf("\n");
return OK;
}
// 在第 i 个位置插入元素 e
Status InsertList(SqList *L, int i, ElemType e) {
if (i < 1 || i > L->sqLength + 1) {
printf("插入位置不合法!");
return ERROR;
}
if (L->sqLength >= MAXSIZE) {
printf("线性表已满,无法插入!");
return ERROR;
}
for (int j = L->sqLength; j >= i; j--) {
L->pList[j] = L->pList[j-1];
}
L->pList[i-1] = e;
L->sqLength++;
return OK;
}
// 删除第 i 个元素,并将其值保存到 e 中
Status DeleteSqList(SqList *L, int i, ElemType *e) {
if (i < 1 || i > L->sqLength) {
printf("删除位置不合法!");
return ERROR;
}
*e = L->pList[i-1];
for (int j = i; j < L->sqLength; j++) {
L->pList[j-1] = L->pList[j];
}
L->sqLength--;
return OK;
}
int main() {
SqList L;
InitList(&L);
CreateList(&L);
PrintList(&L);
InsertList(&L, 3, 99);
PrintList(&L);
ElemType e;
DeleteSqList(&L, 4, &e);
printf("删除的元素为:%d\n", e);
PrintList(&L);
return 0;
}
```
#define MaxSize 100000 #include<stdio.h> #include<stdlib.h> typedef int ElemType; typedef struct{ ElemType data[MaxSize]; int length; }List; void InitList(List &l){ l.length=0; } void swap(int *a,int *b) { int t=*a;*a=*b;*b=t; } int InsElem(List &l,ElemType x,int i){ int j; if(i<1||i>l.length+1) return 0; for(j=l.length;j>i;j--) l.data[j]=l.data[j-1]; l.data[i-1]=x; l.length++; return 1; }
这段代码定义了一个线性表的结构体,并实现了线性表的初始化和插入元素操作。具体解释如下:
1. 宏定义:`#define MaxSize 100000` 定义了线性表最大长度为100000,可以根据实际需求修改。
2. 头文件:`#include<stdio.h>` 和 `#include<stdlib.h>` 分别包含了标准输入输出库和标准库,以便后续使用。
3. 类型定义:`typedef int ElemType;` 定义了一个元素类型为整型的别名 `ElemType`。
4. 结构体定义:`typedef struct{ ElemType data[MaxSize]; int length; }List;` 定义了一个线性表结构体,包含了一个元素数组和当前线性表长度。
5. 初始化函数:`void InitList(List &l){ l.length=0; }` 将线性表的长度设为0,表示该线性表为空。
6. 元素插入函数:`int InsElem(List &l,ElemType x,int i){...}` 将元素 `x` 插入到线性表 `l` 的第 `i` 个位置上,插入成功则返回1,否则返回0。
算法流程:
- 判断插入位置是否合法,若不合法则返回0。
- 从线性表的最后一个元素开始往前遍历,将第 `j` 个元素复制到第 `j+1` 个元素的位置,直到第 `i` 个位置。
- 在第 `i-1` 个位置插入元素 `x`。
- 将线性表长度加1。
- 返回1表示插入成功。
7. 辅助函数:`void swap(int *a,int *b) { int t=*a;*a=*b;*b=t; }` 定义了一个交换两个整型变量值的函数,后续可能会用到。
总的来说,这段代码实现了线性表的初始化和插入元素操作,但是还缺少其它基本操作,如删除元素、查找元素等。如果需要更完善的线性表操作,需要再进行补充。
阅读全文
相关推荐
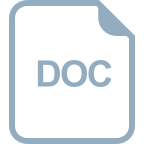
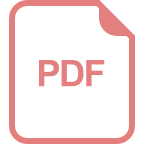
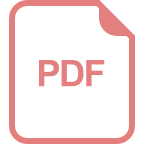












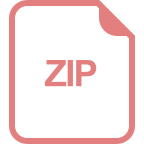
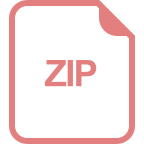
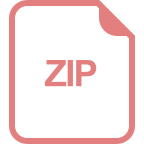