请用C# 写一段从8个bool 值来创建一个字节的代码
时间: 2024-05-06 18:21:51 浏览: 6
语言编写一个函数,接收一个整数数组和它的长度作为参数,计算这个数组中所有元素的和并返回。
以下是示例C代码:
```c
#include <stdio.h>
int sum(int arr[], int len) {
int total = 0;
for (int i = 0; i < len; i++) {
total += arr[i];
}
return total;
}
int main() {
int nums[] = {1, 2, 3, 4, 5};
int len = sizeof(nums) / sizeof(nums[0]);
int result = sum(nums, len);
printf("The sum is %d.\n", result);
return 0;
}
```
在本例中,函数`sum`接收一个整数数组和它的长度作为参数,通过循环计算该数组中所有元素的和并返回。`main`函数中定义了一个整数数组`nums`,以及利用`sizeof`计算数组长度的变量`len`。然后通过调用`sum`函数并传入数组和长度作为参数计算数组中所有元素的和,并将结果输出。
相关问题
用C#写个函数 函数功能是 根据 8个bool 值创建一个字节
语言编程是非常有挑战性的事情,需要有一定的编程经验和技能。C语言是一种比较基础的编程语言,在计算机科学中应用广泛。使用C语言可以进行低级别的系统编程,如操作系统、设备驱动程序和嵌入式系统开发。此外,C语言还可以用于网络编程、游戏开发、算法实现和科学计算等方面。要学好C语言编程,需要掌握基本的语法结构、常用函数和调试技巧。最重要的是,需要有耐心和实践经验,不断学习和提高自己的编程水平。
根据 8个bool 值创建一个字节
把8个bool值存储在一个字节中,可以使用位运算符。
例如,如果我们有以下8个bool值:
bool a = true;
bool b = false;
bool c = true;
bool d = false;
bool e = true;
bool f = false;
bool g = true;
bool h = false;
我们可以将它们组合到一个字节中,使用以下代码:
unsigned char myByte = 0;
myByte |= a << 7;
myByte |= b << 6;
myByte |= c << 5;
myByte |= d << 4;
myByte |= e << 3;
myByte |= f << 2;
myByte |= g << 1;
myByte |= h << 0;
这个代码将每个bool值移位,并将其设置为字节中的相应位。最终结果将是一个8-bit的字节,其中第7位对应于a,第6位对应于b,以此类推。
相关推荐
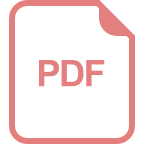
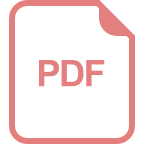
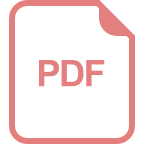












