JAVA通过RJ45接口监控UPS
时间: 2023-12-05 12:03:33 浏览: 223
要通过RJ45接口监控UPS,需要使用Java语言编写一个应用程序,该程序可以与UPS通信并获取UPS的状态信息。
以下是一个使用Java语言监控UPS的示例程序:
1.首先,需要创建一个Java类来代表UPS,该类需要包含UPS的IP地址、端口号、用户名和密码等信息。
public class UPS {
private String ipAddress;
private int port;
private String username;
private String password;
public UPS(String ipAddress, int port, String username, String password) {
this.ipAddress = ipAddress;
this.port = port;
this.username = username;
this.password = password;
}
// Getters and setters
}
2. 接下来,需要创建一个Java类来与UPS通信,该类需要使用Java Socket编程来建立与UPS的连接,并发送和接收数据。
public class UPSConnector {
private Socket socket;
private PrintWriter out;
private BufferedReader in;
public void connect(UPS ups) throws IOException {
socket = new Socket(ups.getIpAddress(), ups.getPort());
out = new PrintWriter(socket.getOutputStream(), true);
in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
// Send login credentials to UPS
out.println(ups.getUsername());
out.println(ups.getPassword());
String response = in.readLine();
if (!response.equals("OK")) {
throw new IOException("Login failed");
}
}
public void disconnect() throws IOException {
if (socket != null) {
socket.close();
}
if (out != null) {
out.close();
}
if (in != null) {
in.close();
}
}
public String sendCommand(String command) throws IOException {
out.println(command);
String response = in.readLine();
return response;
}
}
3. 最后,需要创建一个Java应用程序来启动UPSConnector,并获取UPS的状态信息。
public class UPSMonitor {
public static void main(String[] args) throws IOException {
// Create UPS object with IP address, port, username and password
UPS ups = new UPS("192.168.1.100", 5000, "admin", "password");
// Create UPSConnector object and connect to UPS
UPSConnector connector = new UPSConnector();
connector.connect(ups);
// Send command to UPS to get status information
String status = connector.sendCommand("GET STATUS");
// Display status information
System.out.println("UPS status: " + status);
// Disconnect from UPS
connector.disconnect();
}
}
以上是一个简单的Java程序,可以通过RJ45接口监控UPS。程序连接到UPS,发送命令以获取UPS的状态信息,并在控制台上显示该信息。根据需要,可以修改程序以获取更多的UPS信息,并将其存储到数据库或发送到其他系统。
阅读全文
相关推荐
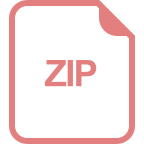
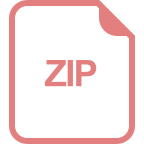
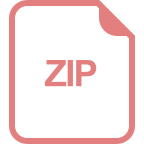
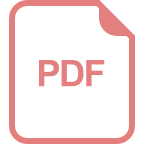
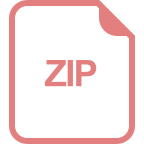
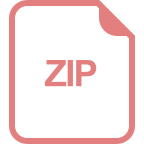
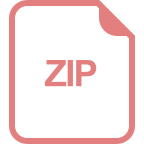
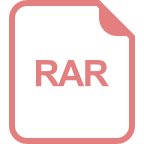