android app 后台到激活回接收到什么消息,怎样用代码处理
时间: 2024-04-12 21:26:41 浏览: 114
当 Android 应用程序从后台到前台激活时,您可以通过以下代码处理接收到的消息:
1. 生命周期回调方法:在活动的生命周期回调方法中处理接收到的消息。例如,在 `onResume()` 方法中处理消息。
```java
@Override
protected void onResume() {
super.onResume();
// 处理接收到的消息
}
```
2. Intent 接收消息:在目标活动中使用 Intent 接收传递的消息。您可以在激活应用程序时的 `onCreate()` 或 `onNewIntent()` 方法中接收消息。
```java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// 获取传递的消息
Intent intent = getIntent();
if (intent != null) {
// 处理接收到的消息
}
}
```
```java
@Override
protected void onNewIntent(Intent intent) {
super.onNewIntent(intent);
// 获取传递的消息
if (intent != null) {
// 处理接收到的消息
}
}
```
3. 广播接收器处理消息:注册广播接收器以接收特定的广播,并在接收到广播时处理消息。您可以在应用程序的 `AndroidManifest.xml` 文件中声明广播接收器,并编写对应的处理代码。
```java
public class MyReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
// 处理接收到的消息
}
}
```
4. 通知处理消息:使用通知机制发送通知,并在用户点击通知时处理消息。您可以通过创建 Notification 对象并使用 NotificationManager 发送通知。
```java
// 创建通知
NotificationCompat.Builder builder = new NotificationCompat.Builder(this, channelId)
.setSmallIcon(R.drawable.notification_icon)
.setContentTitle("Notification Title")
.setContentText("Notification Content")
.setPriority(NotificationCompat.PRIORITY_HIGH);
// 发送通知
NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this);
notificationManager.notify(notificationId, builder.build());
```
在用户点击通知后,您可以在目标活动的 `onCreate()` 或 `onNewIntent()` 方法中接收传递的消息。
以上是一些常见的处理方式示例,具体的实现取决于您的应用程序需求。
阅读全文
相关推荐
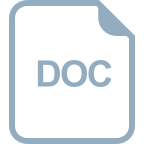
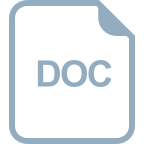
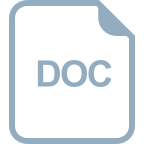

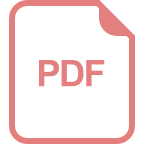
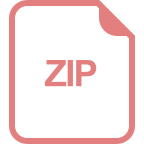
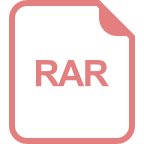
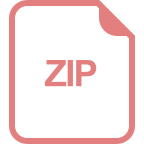
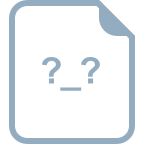
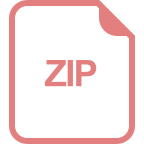
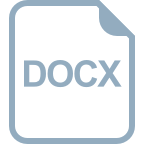
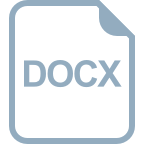
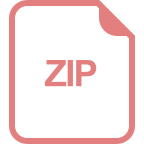
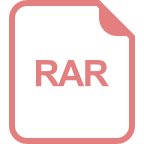
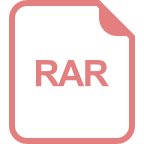
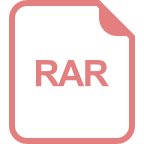
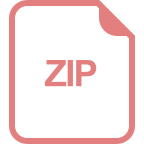
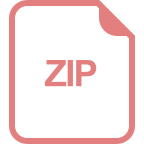
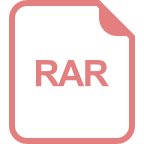