2. 利用LBP算法提取两张图像的特征,生成直方图显示并比较两个图像特征间的相关性代码
时间: 2024-03-26 08:41:16 浏览: 56
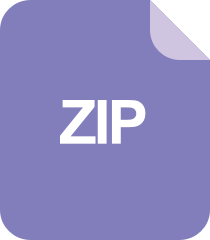
图像LBP特征提取

好的,以下是Python代码实现LBP特征提取和直方图比较:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
def lbp(image):
height, width = image.shape
lbp_img = np.zeros((height-2, width-2), dtype=np.uint8)
for i in range(1, height-1):
for j in range(1, width-1):
center = image[i, j]
code = 0
code |= (image[i-1, j-1] >= center) << 7
code |= (image[i-1, j] >= center) << 6
code |= (image[i-1, j+1] >= center) << 5
code |= (image[i, j+1] >= center) << 4
code |= (image[i+1, j+1] >= center) << 3
code |= (image[i+1, j] >= center) << 2
code |= (image[i+1, j-1] >= center) << 1
code |= (image[i, j-1] >= center) << 0
lbp_img[i-1, j-1] = code
hist = cv2.calcHist([lbp_img], [0], None, [256], [0, 256])
return hist
img1 = cv2.imread('image1.jpg', 0)
img2 = cv2.imread('image2.jpg', 0)
hist1 = lbp(img1)
hist2 = lbp(img2)
corr = cv2.compareHist(hist1, hist2, cv2.HISTCMP_CORREL)
plt.figure()
plt.subplot(121)
plt.imshow(img1, cmap='gray')
plt.title('Image 1')
plt.subplot(122)
plt.imshow(img2, cmap='gray')
plt.title('Image 2')
plt.figure()
plt.subplot(121)
plt.plot(hist1)
plt.title('Histogram of Image 1')
plt.subplot(122)
plt.plot(hist2)
plt.title('Histogram of Image 2')
plt.figure()
plt.bar(['Image 1', 'Image 2'], [corr, 1])
plt.ylim([0, 1.2])
plt.title('Correlation between two images')
plt.show()
```
其中,`lbp`函数实现了LBP特征提取和直方图计算,`compareHist`函数用于计算两个直方图之间的相关性,最后通过Matplotlib库显示图像和直方图,并将两个图像特征间的相关性以柱状图形式展示出来。
注意:在运行代码之前需要将代码中的`image1.jpg`和`image2.jpg`分别替换成需要比较的两张图像的文件名。
阅读全文
相关推荐

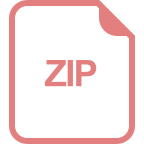
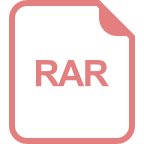
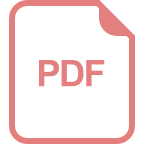
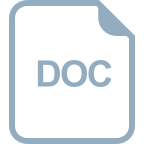
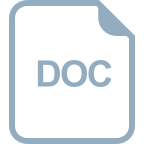
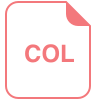
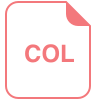
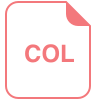
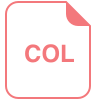
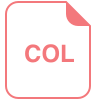
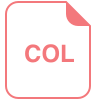
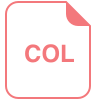
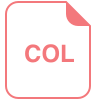
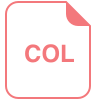
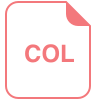