hutool url转base64
时间: 2023-11-25 17:05:06 浏览: 334
以下是Java代码示例:
```java
import cn.hutool.core.codec.Base64;
import cn.hutool.core.util.URLUtil;
public class Test {
public static void main(String[] args) {
String url = "https://www.example.com/image.jpg";
String encodedUrl = Base64.encode(URLUtil.toUrlSafe(url));
System.out.println(encodedUrl);
}
}
```
首先,使用`URLUtil.toUrlSafe`方法将URL转换为URL安全的格式。然后,使用`Base64.encode`方法将URL编码为Base64格式的字符串。最后,打印输出编码后的字符串。
注意,需要引入Hutool的依赖包。可以在Maven中添加以下依赖:
```xml
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.7.6</version>
</dependency>
```
相关问题
hutool 图片转base64
hutool是一款功能强大的Java工具库,可以帮助开发者快速便捷地进行各种常见的操作。其中,hutool也提供了图片转base64的功能。
要使用hutool实现图片转base64的功能,首先需要导入hutool的相关依赖包。然后,通过以下步骤来完成图片转base64的操作:
1. 使用hutool的`ImageUtil`类的`read`方法,将图片文件读取为`BufferedImage`对象。例如,可以使用`BufferedImage image = ImageUtil.read(图片文件路径)`来读取一张图片。
2. 使用hutool的`Base64Encoder`类,将读取到的`BufferedImage`对象转换为base64字符串。例如,可以使用`String base64Str = Base64Encoder.encode(image, Format.PNG)`来将`image`对象转换为PNG格式的base64字符串。
3. 如果需要对base64字符串进行进一步处理,可以使用hutool的`StrUtil`类进行字符串操作。例如,可以使用`StrUtil.removePrefix(base64Str, "data:image/png;base64,")`将base64字符串中的前缀"data:image/png;base64,"移除。
通过以上步骤,我们就可以使用hutool实现图片转base64的功能了。使用hutool进行图片转base64的好处是,代码简洁、易用,并且hutool还提供了许多其他功能,可以帮助开发者更高效地完成各种任务。
hutool5.8.12 url转base64
在Hutool 5.8.12中,可以使用Base64Encoder类将URL转换为Base64编码。以下是示例代码:
```java
import cn.hutool.core.codec.Base64Encoder;
public class UrlToBase64Example {
public static void main(String[] args) {
String url = "https://www.example.com/image.png";
String base64 = Base64Encoder.encodeUrlSafe(url.getBytes());
System.out.println(base64);
}
}
```
在上面的示例中,我们将URL https://www.example.com/image.png 转换为Base64编码,使用了Base64Encoder.encodeUrlSafe() 方法。该方法将URL转换为Base64编码,并且使用URL安全的字符集,避免在传输过程中出现问题。最后,我们将Base64编码打印到控制台上。
输出结果应该为:aHR0cHM6Ly93d3cuZXhhbXBsZS5jb20vaW1hZ2UucG5n
注意:使用Base64编码时,输出的结果长度会比原始数据长度更长,这是因为Base64编码需要将每3个字节转换为4个字节,因此会产生额外的填充字符。
相关推荐
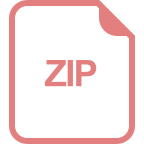












