商城后台管理系统点赞代码
时间: 2024-12-30 17:22:37 浏览: 4
### 商城后台管理系统中的点赞功能实现
#### 功能需求分析
在商城后台管理系统中,点赞功能允许用户对特定的商品、文章或其他内容进行评价。这一机制不仅能够提升用户体验,还能帮助管理员了解哪些产品更受欢迎。为了实现该功能,需考虑前端交互以及后端数据处理两方面。
#### 前端设计与开发
对于前端而言,在Vue框架下可以通过`axios`库发起HTTP请求完成点赞操作。当用户点击某个商品对应的赞按钮时,触发事件处理器发送AJAX请求给服务器并更新UI显示当前状态(已赞/未赞)。此外还需注意防抖动措施以防止短时间内多次提交相同请求造成不必要的资源浪费[^1]。
```javascript
// Vue组件方法内定义点赞函数
methods: {
async likeProduct(productId) {
try {
const response = await axios.post('/api/products/' + productId + '/like');
console.log(response.data);
this.$message.success('点赞成功!');
// 更新本地视图状态
let productIndex = this.products.findIndex(item => item.id === productId);
if (productIndex !== -1){
this.products[productIndex].likedCount += 1;
}
} catch(error) {
console.error("Error:", error);
this.$message.error('点赞失败,请稍后再试...');
}
}
}
```
#### 后端接口搭建
采用Spring Boot构建RESTful API用于接收来自客户端的点赞请求,并将其保存至数据库中。考虑到安全性问题,应当验证用户的登录身份信息,确保只有合法注册过的成员才能执行此动作;同时为了避免重复投票现象的发生,建议引入唯一约束条件或者记录每次行为的时间戳以便后续审计追踪。
```java
@RestController
@RequestMapping("/api/products")
public class ProductController {
@Autowired
private ProductService productService;
/**
* 处理POST /products/{id}/like路径下的点赞请求
*/
@PostMapping("/{id}/like")
public ResponseEntity<String> handleLikeRequest(@PathVariable Long id, HttpServletRequest request) throws Exception{
String token = JwtTokenUtil.resolveToken(request); // 获取JWT令牌
if (!JwtTokenUtil.validateToken(token)){
throw new UnauthorizedException("Invalid Token");
}
Long userId = JwtTokenUtil.getUserIdFromToken(token);
boolean result = productService.like(id, userId);
return result ? ResponseEntity.ok().body("Liked successfully") : ResponseEntity.badRequest().build();
}
}
```
#### 数据库表结构设计
针对上述业务场景,可以创建一张名为`likes`的新表格用来存储具体的点赞关系。其中至少应包含三个字段:`user_id`(关联到会员表),`target_type`(表示目标实体类别如'PRODUCT') 和 `target_id`(对应的目标ID)。
```sql
CREATE TABLE likes (
user_id BIGINT NOT NULL,
target_type VARCHAR(50),
target_id BIGINT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY(user_id,target_type,target_id), /* 组合键保证单个用户对同一对象仅能有一次有效点赞 */
FOREIGN KEY (user_id) REFERENCES users(id) ON DELETE CASCADE
);
```
阅读全文
相关推荐


















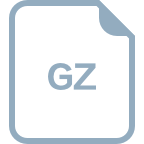