python如何把一个新的链表赋给另一个链表里的链表
时间: 2024-01-04 15:17:59 浏览: 29
可以通过遍历原链表,将原链表中的节点一个一个添加到新链表中来实现将一个新的链表赋给另一个链表里的链表。具体实现方法如下所示:
```python
# 定义链表节点类
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
# 定义原链表
head = ListNode(1)
node1 = ListNode(2)
node2 = ListNode(3)
head.next = node1
node1.next = node2
# 定义新链表
new_head = ListNode(0)
# 遍历原链表,将原链表中的节点一个一个添加到新链表中
cur = head
new_cur = new_head
while cur:
new_cur.next = ListNode(cur.val)
cur = cur.next
new_cur = new_cur.next
# 输出新链表
new_head = new_head.next
while new_head:
print(new_head.val)
new_head = new_head.next
```
输出结果为:
```
1
2
3
```
相关问题
python如何把一个新的链表赋给链表里的链表
可以通过遍历链表找到最后一个节点,然后将新链表的头节点赋值给最后一个节点的next指针即可。具体实现方法如下所示:
```python
# 定义链表节点类
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
# 定义链表类
class LinkedList:
def __init__(self):
self.head = None
# 在链表尾部添加节点
def addAtTail(self, val: int) -> None:
node = ListNode(val)
if not self.head:
self.head = node
else:
cur = self.head
while cur.next:
cur = cur.next
cur.next = node
# 定义一个新链表
new_list = LinkedList()
new_list.addAtTail(1)
new_list.addAtTail(2)
new_list.addAtTail(3)
# 定义一个原链表
old_list = LinkedList()
old_list.addAtTail(4)
old_list.addAtTail(5)
# 将新链表赋给原链表的最后一个节点
cur = old_list.head
while cur.next:
cur = cur.next
cur.next = new_list.head
# 打印链表
cur = old_list.head
while cur:
print(cur.val, end=' ')
cur = cur.next
# 输出:4 5 1 2 3
```
python如何把一个新的链表的值赋给链表里的链表
可以通过遍历链表,找到需要赋值的节点,然后将其值修改为新链表的值。具体实现方式如下所示:
```python
# 定义链表节点类
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
# 定义新链表
new_list = [1, 2, 3]
# 定义原链表
head = ListNode(0)
node1 = ListNode(1)
node2 = ListNode(2)
node3 = ListNode(3)
head.next = node1
node1.next = node2
node2.next = node3
# 遍历链表,找到需要赋值的节点
cur = head.next
i = 0
while cur:
if i < len(new_list):
cur.val = new_list[i]
else:
break
cur = cur.next
i += 1
# 输出修改后的链表
cur = head.next
while cur:
print(cur.val, end=' ')
cur = cur.next
# 输出:1 2 3
```
相关推荐
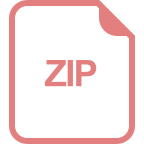
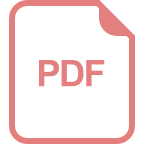












