uni-app实现点赞评论功能
时间: 2023-10-02 17:05:46 浏览: 175
要实现点赞评论功能,您需要进行以下步骤:
1. 创建一个页面用于显示评论和点赞功能。
2. 在页面中添加一个表单用于输入评论内容。
3. 在页面中添加一个列表用于显示评论内容和点赞数量。
4. 在列表项中添加点赞按钮。
5. 在点击点赞按钮时,向后端发送请求更新点赞数量。
6. 在点击提交按钮时,向后端发送请求添加评论并更新列表。
下面是一些示例代码:
1. 创建页面
// pages/comment.vue
<template>
<div>
<form @submit.prevent="submitComment">
<textarea v-model="comment"></textarea>
<button type="submit">提交</button>
</form>
<ul>
<li v-for="item in comments" :key="item.id">
<p>{{ item.comment }}</p>
<button @click="likeComment(item.id)">点赞 {{ item.likes }}</button>
</li>
</ul>
</div>
</template>
<script>
import { mapActions, mapGetters } from 'vuex'
export default {
computed: {
...mapGetters(['comments'])
},
data() {
return {
comment: ''
}
},
methods: {
...mapActions(['addComment', 'likeComment']),
submitComment() {
this.addComment(this.comment)
this.comment = ''
}
}
}
</script>
2. Vuex 状态管理
// store/index.js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
comments: []
},
mutations: {
SET_COMMENTS(state, comments) {
state.comments = comments
},
ADD_COMMENT(state, comment) {
state.comments.push(comment)
},
LIKE_COMMENT(state, id) {
const comment = state.comments.find(item => item.id === id)
comment.likes++
}
},
actions: {
async fetchComments({ commit }) {
const res = await fetch('/api/comments')
const comments = await res.json()
commit('SET_COMMENTS', comments)
},
async addComment({ commit }, comment) {
const res = await fetch('/api/comments', {
method: 'POST',
body: JSON.stringify({ comment }),
headers: { 'Content-Type': 'application/json' }
})
const newComment = await res.json()
commit('ADD_COMMENT', newComment)
},
async likeComment({ commit }, id) {
await fetch(`/api/comments/${id}/like`, { method: 'POST' })
commit('LIKE_COMMENT', id)
}
},
getters: {
comments: state => state.comments
}
})
3. 后端接口
// server.js
const express = require('express')
const app = express()
const comments = []
app.use(express.json())
app.get('/api/comments', (req, res) => {
res.json(comments)
})
app.post('/api/comments', (req, res) => {
const id = comments.length + 1
const comment = { id, comment: req.body.comment, likes: 0 }
comments.push(comment)
res.json(comment)
})
app.post('/api/comments/:id/like', (req, res) => {
const id = parseInt(req.params.id, 10)
const comment = comments.find(item => item.id === id)
if (comment) {
comment.likes++
res.sendStatus(200)
} else {
res.sendStatus(404)
}
})
app.listen(3000, () => {
console.log('Server started on http://localhost:3000')
})
4. 页面初始化
// main.js
import Vue from 'vue'
import App from './App.vue'
import store from './store'
Vue.config.productionTip = false
new Vue({
store,
beforeCreate() {
this.$store.dispatch('fetchComments')
},
render: h => h(App)
}).$mount('#app')
阅读全文
相关推荐
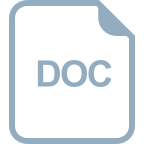
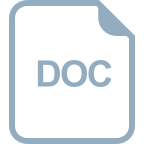
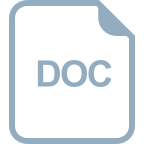

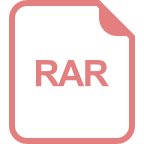
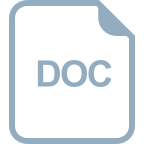

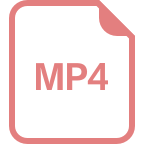
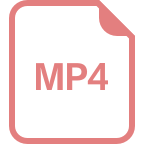
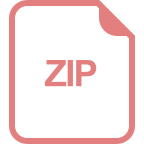
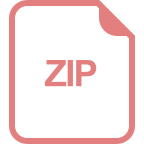
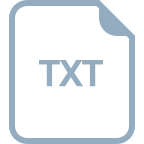
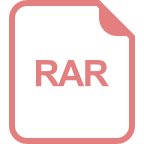



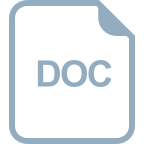
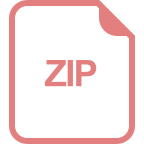
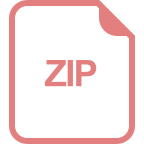
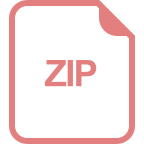