Please define a class Book and its data members and member functions ,according to the following description.Don’t implement any member functions. (1) Each book has a title, one or more authors, and an international standard book number (ISBN). (2) Class Book has two constructor: (a) the first should be a default constructor, (b) the second should take two arguments: the title and the ISBN. (3) The authors can be added one by one. (4) For the title and ISBN of a Book,a “set”function and a “get” function are required,Each “set ”function undates the corresponding data member to a new value through its parameters and each “get”function returns the value of the corresponding data member to caller.
时间: 2024-03-25 18:35:51 浏览: 95
Here is an example implementation of the Book class:
```c++
class Book {
private:
string title; // the book title
vector<string> authors; // the book authors
string isbn; // the book ISBN
public:
// Default constructor
Book() {
title = "";
isbn = "";
}
// Constructor with title and ISBN arguments
Book(string title, string isbn) {
this->title = title;
this->isbn = isbn;
}
// Add an author to the vector of authors
void addAuthor(string author) {
authors.push_back(author);
}
// Set the book title
void setTitle(string title) {
this->title = title;
}
// Set the book ISBN
void setISBN(string isbn) {
this->isbn = isbn;
}
// Get the book title
string getTitle() const {
return title;
}
// Get the book ISBN
string getISBN() const {
return isbn;
}
};
```
In this implementation, the Book class has three private data members: `title`, `authors`, and `isbn`. It also has a default constructor, which sets `title` and `isbn` to empty strings, and a constructor that takes `title` and `isbn` arguments.
The `addAuthor` function allows new authors to be added to the vector of authors. The `setTitle` and `setISBN` functions update the corresponding data members with new values passed in through their parameters.
Finally, there are two "get" functions: `getTitle` and `getISBN`, which return the values of the corresponding data members. These functions are marked as `const` to indicate that they do not modify the object.
阅读全文
相关推荐
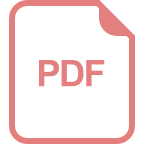
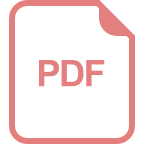
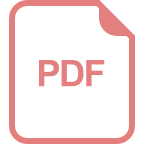
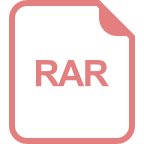
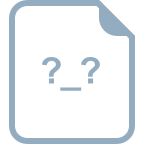
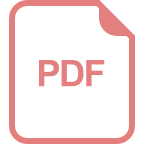
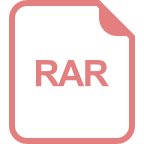
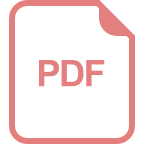
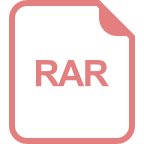
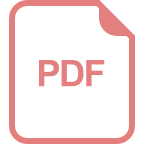
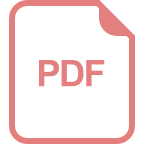
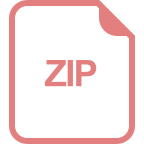
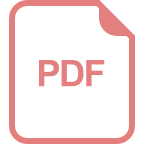
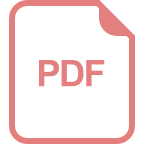
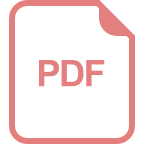
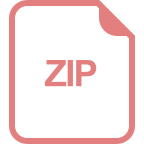
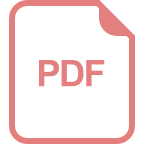
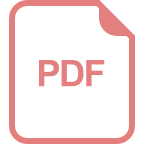