在linux添加一个系统调用, 该系统调用接受两个参数:参数1:以整型数表示的自己学号的后3位;参数2:flag,取值为0或1,若为0,该系统调用的返回值为参数1的个位。若为1。该系统调用的返回值为参数1的十位。 2. 对上面的系统调用进行修改,修改如下: i. 声明一个内核全局变量gOSE, 该系统首先将参数1的值赋给gOSE,并且,对于参数2:flag,取值为0或1,若为0,将gOSE的值按位取反,后赋值给gOSE,然后返回gOSE的值;若为1,则将gOSE的值与0Xffffffff异或,后赋值给gOSE,然后返回gOSE的值; ii. 加入内核互斥锁,使得两个进程在调用该系统调用时,能够做到互斥访问gOSE。 2. 对于系统调用编程题中的strict alternation算法,给出该算法中turn变量的虚地址,并且给出该变量的物理地址。,给出代码
时间: 2023-12-11 16:05:57 浏览: 100
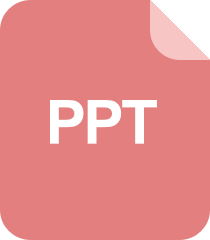
linux中添加系统调用
对于第一个问题,你需要了解Linux内核编程相关知识。下面是一个简单的实现:
首先,在Linux内核源码目录下的arch/x86/entry/syscalls/syscall_64.tbl文件中添加一行:
400 common mysyscall __x64_sys_mysyscall
然后,在include/linux/syscalls.h文件中添加以下内容:
asmlinkage long sys_mysyscall(int num, int flag);
接下来,在kernel/sys.c文件中实现sys_mysyscall函数:
asmlinkage long sys_mysyscall(int num, int flag)
{
int ret = 0;
if (flag == 0) {
ret = num % 10;
} else if (flag == 1) {
ret = (num / 10) % 10;
}
return ret;
}
最后,在Makefile文件中添加以下内容:
obj-y += mysyscall.o
这样就可以编译内核并启用该系统调用了。使用时,可以通过调用syscall函数来调用mysyscall系统调用。
对于第二个问题,strict alternation算法中的turn变量的虚地址是一个指针,指向一个整型变量。该变量的物理地址需要通过调试器或者其他工具获取,因为每次内存分配的位置都可能不同。以下是一个简单的strict alternation算法的实现:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
int turn = 0;
int *p_turn;
void *thread_func(void *arg)
{
int id = *(int *)arg;
int i;
for (i = 0; i < 10; i++) {
while (*p_turn != id) {
usleep(1000);
}
printf("Thread %d: %d\n", id, i);
*p_turn = (id + 1) % 2;
}
return NULL;
}
int main()
{
pthread_t t1, t2;
int id1 = 0, id2 = 1;
p_turn = &turn;
*p_turn = 0;
pthread_create(&t1, NULL, thread_func, &id1);
pthread_create(&t2, NULL, thread_func, &id2);
pthread_join(t1, NULL);
pthread_join(t2, NULL);
return 0;
}
阅读全文
相关推荐









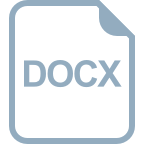
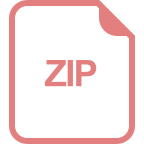