编写java程序代码实现一个常见的加解密算法的可交互的程序
时间: 2023-06-24 11:08:23 浏览: 106
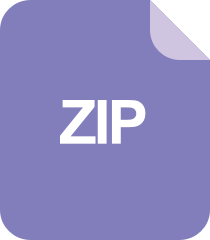
RSA:一个独立的 Java 桌面应用程序从头开始实现 RSA 加密算法
以下是一个使用Java实现常用加解密算法的可交互程序,包括AES、DES、RSA和MD5算法:
```java
import java.security.*;
import javax.crypto.*;
import javax.crypto.spec.*;
import java.math.*;
import java.util.*;
public class EncryptionProgram {
public static void main(String[] args) throws Exception {
Scanner sc = new Scanner(System.in);
System.out.println("Welcome to the Encryption Program!\n");
while (true) {
System.out.println("Please choose an encryption algorithm:");
System.out.println("1. AES");
System.out.println("2. DES");
System.out.println("3. RSA");
System.out.println("4. MD5");
System.out.println("5. Exit");
int choice = sc.nextInt();
switch (choice) {
case 1:
aesEncryption();
break;
case 2:
desEncryption();
break;
case 3:
rsaEncryption();
break;
case 4:
md5Encryption();
break;
case 5:
System.out.println("Goodbye!");
sc.close();
System.exit(0);
default:
System.out.println("Invalid choice!\n");
break;
}
}
}
public static void aesEncryption() throws Exception {
Scanner sc = new Scanner(System.in);
System.out.println("\nAES Encryption\n");
System.out.print("Enter the message you want to encrypt: ");
String message = sc.nextLine();
System.out.print("Enter the secret key: ");
String secretKey = sc.nextLine();
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
SecureRandom random = new SecureRandom(secretKey.getBytes());
keyGen.init(random);
SecretKey key = keyGen.generateKey();
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encrypted = cipher.doFinal(message.getBytes());
System.out.println("Encrypted message: " + new String(encrypted));
}
public static void desEncryption() throws Exception {
Scanner sc = new Scanner(System.in);
System.out.println("\nDES Encryption\n");
System.out.print("Enter the message you want to encrypt: ");
String message = sc.nextLine();
System.out.print("Enter the secret key: ");
String secretKey = sc.nextLine();
DESKeySpec keySpec = new DESKeySpec(secretKey.getBytes());
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES");
SecretKey key = keyFactory.generateSecret(keySpec);
Cipher cipher = Cipher.getInstance("DES");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encrypted = cipher.doFinal(message.getBytes());
System.out.println("Encrypted message: " + new String(encrypted));
}
public static void rsaEncryption() throws Exception {
Scanner sc = new Scanner(System.in);
System.out.println("\nRSA Encryption\n");
System.out.print("Enter the message you want to encrypt: ");
String message = sc.nextLine();
KeyPairGenerator keyGen = KeyPairGenerator.getInstance("RSA");
keyGen.initialize(1024);
KeyPair keyPair = keyGen.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encrypted = cipher.doFinal(message.getBytes());
System.out.println("Encrypted message: " + new String(encrypted));
}
public static void md5Encryption() throws Exception {
Scanner sc = new Scanner(System.in);
System.out.println("\nMD5 Encryption\n");
System.out.print("Enter the message you want to encrypt: ");
String message = sc.nextLine();
MessageDigest md = MessageDigest.getInstance("MD5");
byte[] messageDigest = md.digest(message.getBytes());
BigInteger no = new BigInteger(1, messageDigest);
String hashtext = no.toString(16);
while (hashtext.length() < 32) {
hashtext = "0" + hashtext;
}
System.out.println("Encrypted message: " + hashtext);
}
}
```
在这个程序中,用户可以选择要使用的加密算法,并输入要加密的消息和秘钥。然后程序将对消息执行加密,并输出加密后的结果。对于MD5算法,输出的是哈希值。
此程序仅用于演示目的,实际应用中需要进行更多的错误处理和异常处理。
阅读全文
相关推荐
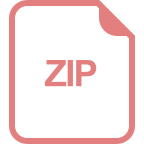
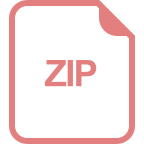















