c#使用httpclient实现wsdl接口上传
时间: 2024-10-24 15:16:44 浏览: 31
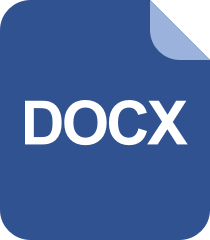
httpClient调用webservice接口

在C#中,你可以使用HttpClient类配合WSDL(Web Services Description Language)文件来调用并上传数据到基于SOAP(Simple Object Access Protocol)的服务。以下是一个基本步骤:
1. **添加命名空间和引用**:
首先,你需要添加`System.Net.Http`和`System.Xml.Linq`命名空间,它们包含了HttpClient和Linq-to-XML的支持。
```csharp
using System;
using System.Net.Http;
using System.Xml.Linq;
```
2. **加载WSDL文件**:
使用`XDocument`加载WSDL文件,获取服务地址和其他信息。
```csharp
string wsdlUrl = "your.wsdl";
XDocument doc = XDocument.Load(wsdlUrl);
var serviceEndpoint = doc.Root.Element("service").Element("port").Attribute("address").Value;
```
3. **创建HttpClient实例**:
创建一个HttpClient实例,用于发起HTTP请求。
```csharp
HttpClient client = new HttpClient();
```
4. **构建SOAP消息**:
根据WSDL生成SOAP消息,这通常涉及到构造一个`XmlWriter`或字符串,并设置合适的SOAP头部和主体(包括上传的数据)。
```csharp
string soapMessage =
@"<?xml version=""1.0"" encoding=""utf-8""?>
<soap:Envelope xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" xmlns:xsd=""http://www.w3.org/2001/XMLSchema"" xmlns:soap=""http://schemas.xmlsoap.org/soap/envelope/"">
<soap:Body>
<YourOperation xmlns=""YourNamespace"">
<!-- Your data goes here -->
</YourOperation>
</soap:Body>
</soap:Envelope>";
```
5. **发送POST请求**:
设置POST请求,将SOAP消息作为Content。
```csharp
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Post, serviceEndpoint);
request.Content = new StringContent(soapMessage, Encoding.UTF8, "text/xml");
```
6. **处理响应**:
发送请求并捕获返回结果。注意,SOAP服务可能会返回XML响应,可以解析它获取数据。
```csharp
HttpResponseMessage response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
// Parse the XML response as needed
XDocument responseData = XDocument.Parse(await response.Content.ReadAsStringAsync());
```
7. **错误处理**:
检查请求是否成功以及可能出现的任何异常。
```csharp
if (response.IsSuccessStatusCode)
{
// Handle success case
}
else
{
Console.WriteLine($"Error: {response.StatusCode} - {await response.Content.ReadAsStringAsync()}");
}
```
阅读全文
相关推荐
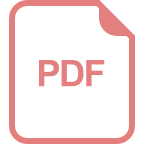
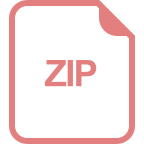















