用Java语言编写贪心算法求解图的最短回路
时间: 2023-08-04 07:30:14 浏览: 49
以下是使用Java语言编写贪心算法求解图的最短回路的代码:
```java
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Comparator;
import java.util.PriorityQueue;
public class ShortestCircuit {
private int V;
private ArrayList<ArrayList<Node>> adjList;
public ShortestCircuit(int V) {
this.V = V;
adjList = new ArrayList<>();
for (int i = 0; i < V; i++) {
adjList.add(new ArrayList<>());
}
}
public void addEdge(int u, int v, int w) {
adjList.get(u).add(new Node(v, w));
adjList.get(v).add(new Node(u, w));
}
public int shortestCircuit() {
int[] dist = new int[V];
Arrays.fill(dist, Integer.MAX_VALUE);
PriorityQueue<Node> pq = new PriorityQueue<>(Comparator.comparingInt(node -> node.weight));
// Set distance of source vertex to 0
dist[0] = 0;
pq.offer(new Node(0, 0));
while (!pq.isEmpty()) {
Node curr = pq.poll();
int u = curr.vertex;
for (Node neighbor : adjList.get(u)) {
int v = neighbor.vertex;
int w = neighbor.weight;
if (dist[v] > dist[u] + w) {
dist[v] = dist[u] + w;
pq.offer(new Node(v, dist[v]));
}
}
}
int minCircuit = Integer.MAX_VALUE;
for (int u = 0; u < V; u++) {
for (Node neighbor : adjList.get(u)) {
int v = neighbor.vertex;
int w = neighbor.weight;
if (dist[u] != Integer.MAX_VALUE && dist[v] != Integer.MAX_VALUE && minCircuit > dist[u] + w + dist[v]) {
minCircuit = dist[u] + w + dist[v];
}
}
}
return minCircuit;
}
private static class Node {
int vertex;
int weight;
public Node(int vertex, int weight) {
this.vertex = vertex;
this.weight = weight;
}
}
public static void main(String[] args) {
ShortestCircuit g = new ShortestCircuit(4);
g.addEdge(0, 1, 10);
g.addEdge(1, 2, 20);
g.addEdge(2, 3, 30);
g.addEdge(3, 0, 40);
int minCircuit = g.shortestCircuit();
System.out.println("Minimum circuit length: " + minCircuit);
}
}
```
在这个实现中,我们使用了优先队列来存储节点,以便按照距离顺序处理它们。我们还使用了邻接表来存储图形。对于每个节点,我们计算它到源节点的距离,并将其添加到优先队列中。然后,我们遍历所有边,计算每个环的长度,并返回最短环的长度。
相关推荐
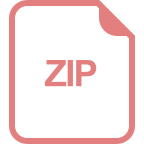
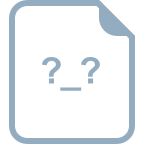














