如何在.ini配置文件中定义一个集合,并用python遍历调用
时间: 2024-11-28 22:27:15 浏览: 26
在`.ini`配置文件中,通常用于存储键值对,不适合直接定义集合这种数据结构,因为`.ini`文件更适用于文本形式的简单配置。然而,如果你需要存储类似列表或字典的数据,可以将其转换成字符串并按照一定的格式编写,比如:
```ini
; 示例 ini 文件
[my_section]
elements = element1,element2,element3
```
在这个例子中,`elements`是一个字符串,它包含逗号分隔的元素,可以视为一个列表。
在Python中读取和处理这样的 `.ini` 文件时,你可以使用 `configparser` 模块:
```python
import configparser
# 读取ini文件
config = configparser.ConfigParser()
config.read('example.ini')
# 将 elements 字段解析为 list
elements_list = config.get('my_section', 'elements').split(',')
# 遍历元素列表
for element in elements_list:
print(f"元素:{element}")
相关问题
python读取ini配置文件# 定义文件路径 path = r'D:\Python_Script\new_framework\source_file\broswer_config.ini'
在Python中,你可以使用`configparser`模块来读取INI格式的配置文件。这个模块提供了一种简单的方式来解析和操作INIs。下面是使用它读取`browser_config.ini`文件的一个示例:
```python
import configparser
# 定义文件路径
config_path = r"D:\Python_Script\new_framework\source_file\browser_config.ini"
# 创建ConfigParser实例
config = configparser.ConfigParser()
# 使用read()方法加载配置文件
config.read(config_path)
# 读取键值对
section_name = 'Section1' # 假设这是你的配置节名
key = 'username'
value = config.get(section_name, key)
print(f"从 {section_name} 节获取的用户名是: {value}")
# 更进一步,可以遍历整个文件
for section in config.sections():
for option, value in config.items(section):
print(f"{section}: {option} = {value}")
```
在这个例子中,我们首先导入了`configparser`模块,然后设置了`config_path`变量指向你的ini文件。通过`ConfigParser()`创建了一个`ConfigParser`对象,接着调用`config.read()`方法读取配置文件。最后,我们可以通过`get()`方法按节和键获取具体的配置项。
如何遍历读取配置文件中的内容
在 Python 中,可以使用 ConfigParser 模块来读取配置文件。以下是一个示例代码:
```python
import configparser
config = configparser.ConfigParser()
config.read('config.ini') # 读取配置文件
# 获取所有的 section
sections = config.sections()
for section in sections:
print(section)
# 获取指定 section 中的所有配置项
options = config.options('database')
for option in options:
print(option, config.get('database', option))
# 获取指定 section 中的所有键值对
items = config.items('database')
for item in items:
print(item[0], item[1])
```
在上面的示例中,我们首先创建了一个 ConfigParser 对象,并调用了它的 read() 方法来读取配置文件。然后,通过调用 sections() 方法获取所有的 section,options() 方法获取指定 section 中的所有配置项,以及 items() 方法获取指定 section 中的所有键值对。最后,我们通过循环遍历打印出了每个 section、配置项和键值对的内容。
阅读全文
相关推荐







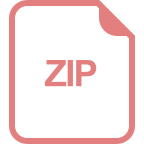



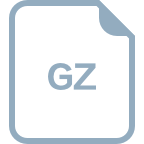




