HttpClient发送Post请求并携带cookie
时间: 2023-05-28 07:05:40 浏览: 103
以下是一个使用HttpClient发送Post请求并携带cookie的示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.CookieStore;
import org.apache.http.client.HttpClient;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.cookie.Cookie;
import org.apache.http.impl.client.BasicCookieStore;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import java.util.ArrayList;
import java.util.List;
public class HttpClientUtil {
public static void main(String[] args) throws Exception {
// 创建HttpClient实例
HttpClient httpClient = HttpClientBuilder.create().build();
// 创建CookieStore实例
CookieStore cookieStore = new BasicCookieStore();
// 创建HttpPost实例
HttpPost httpPost = new HttpPost("http://example.com/login");
// 设置请求参数
RequestConfig requestConfig = RequestConfig.custom()
.setConnectTimeout(5000).setConnectionRequestTimeout(5000)
.setSocketTimeout(5000).build();
httpPost.setConfig(requestConfig);
// 设置请求头
httpPost.setHeader("Content-Type", "application/x-www-form-urlencoded");
// 设置请求体参数
List<BasicNameValuePair> parameters = new ArrayList<>();
parameters.add(new BasicNameValuePair("username", "example"));
parameters.add(new BasicNameValuePair("password", "password"));
UrlEncodedFormEntity formEntity = new UrlEncodedFormEntity(parameters);
httpPost.setEntity(formEntity);
// 执行HttpPost请求
HttpResponse httpResponse = httpClient.execute(httpPost);
// 获取响应实体
HttpEntity httpEntity = httpResponse.getEntity();
if (httpEntity != null) {
String response = EntityUtils.toString(httpEntity);
System.out.println(response);
}
// 获取Cookie
List<Cookie> cookies = cookieStore.getCookies();
// 创建HttpPost实例
httpPost = new HttpPost("http://example.com/data");
// 设置请求参数
requestConfig = RequestConfig.custom()
.setConnectTimeout(5000).setConnectionRequestTimeout(5000)
.setSocketTimeout(5000).build();
httpPost.setConfig(requestConfig);
// 设置请求头
httpPost.setHeader("Content-Type", "application/json");
httpPost.setHeader("Accept", "application/json");
// 设置请求体参数
String requestBody = "{\"key\":\"value\"}";
httpPost.setEntity(new StringEntity(requestBody, ContentType.APPLICATION_JSON));
// 设置Cookie
httpClient = HttpClientBuilder.create().setDefaultCookieStore(cookieStore).build();
// 执行HttpPost请求
httpResponse = httpClient.execute(httpPost);
// 获取响应实体
httpEntity = httpResponse.getEntity();
if (httpEntity != null) {
String response = EntityUtils.toString(httpEntity);
System.out.println(response);
}
}
}
```
相关推荐
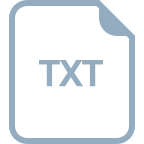
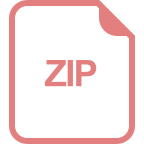












