用C++调用命令行解释器,执行一条命令,并将执行结果封装在一个名为cmdRet的数据结构中。
时间: 2023-05-20 08:06:27 浏览: 52
可以使用system函数来调用命令行解释器执行命令,然后将执行结果存储在一个字符数组中。以下是一个示例代码:
```
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#define MAX_CMD_LEN 1024
#define MAX_RET_LEN 1024
typedef struct {
int retCode;
char retMsg[MAX_RET_LEN];
} cmdRet;
cmdRet executeCmd(char* cmd) {
cmdRet ret;
char buffer[MAX_RET_LEN];
FILE* pipe = popen(cmd, "r");
if (!pipe) {
ret.retCode = -1;
strcpy(ret.retMsg, "Failed to execute command");
return ret;
}
while (!feof(pipe)) {
if (fgets(buffer, MAX_RET_LEN, pipe) != NULL) {
strcat(ret.retMsg, buffer);
}
}
ret.retCode = pclose(pipe);
return ret;
}
int main() {
char cmd[MAX_CMD_LEN];
printf("Enter command to execute: ");
fgets(cmd, MAX_CMD_LEN, stdin);
cmdRet ret = executeCmd(cmd);
printf("Return code: %d\n", ret.retCode);
printf("Return message: %s\n", ret.retMsg);
return 0;
}
```
在上面的代码中,executeCmd函数接受一个命令字符串作为参数,使用popen函数打开一个管道来执行命令,并将执行结果存储在一个字符数组中。最后,函数返回一个cmdRet结构体,其中包含命令的返回代码和返回消息。
在主函数中,我们首先从用户输入中获取要执行的命令,然后调用executeCmd函数来执行命令并获取结果。最后,我们打印出返回代码和返回消息。
请注意,这只是一个简单的示例代码,实际情况可能需要更多的错误处理和安全性检查。
相关推荐
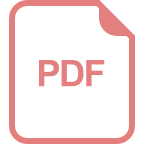














