用Python写一个俄罗斯方块游戏
时间: 2023-03-14 11:41:27 浏览: 41
很高兴为您提供帮助。对于用Python写一个俄罗斯方块游戏,首先要确定游戏的基本结构,然后定义游戏的规则,并根据规则开发游戏算法。然后,使用Python语言进行编程,实现游戏的功能。最后,进行调试,使游戏能够正常运行。
相关问题
用python写一个俄罗斯方块游戏
好的,下面是一个简单的俄罗斯方块游戏的代码示例,基于Python的pygame库实现:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口尺寸
WIDTH, HEIGHT = 600, 700
WINDOW = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("俄罗斯方块")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义方块大小和间隙
BLOCK_SIZE = 30
BLOCK_GAP = 5
# 定义方块形状和颜色
SHAPES = [
[[1, 1, 1], [0, 1, 0]],
[[2, 2, 0], [0, 2, 2]],
[[3, 3, 3, 3]],
[[4, 4], [4, 4]],
[[0, 5, 5], [5, 5, 0]],
[[6, 6, 6], [6, 0, 0]],
[[7, 7, 7], [0, 0, 7]]
]
COLORS = [
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(192, 192, 192)
]
# 定义游戏区域
PLAY_WIDTH, PLAY_HEIGHT = 10 * BLOCK_SIZE, 20 * BLOCK_SIZE
PLAY_AREA = pygame.Rect(0, 0, PLAY_WIDTH, PLAY_HEIGHT)
# 定义字体
FONT = pygame.font.SysFont("Arial", 30)
# 定义游戏状态
RUNNING = "running"
GAME_OVER = "game over"
# 定义游戏速度
FPS = 60
SPEED = 500
# 定义方块类
class Block:
def __init__(self, x, y, shape):
self.x = x
self.y = y
self.shape = shape
self.color = COLORS[SHAPES.index(self.shape)]
self.rotation = 0
# 顺时针旋转方块
def rotate(self):
self.rotation = (self.rotation + 1) % len(self.shape)
# 获取当前方块的形状
def get_shape(self):
return self.shape[self.rotation]
# 获取方块的位置
def get_position(self):
return self.x, self.y
# 设置方块的位置
def set_position(self, x, y):
self.x = x
self.y = y
# 绘制方块
def draw(self, surface):
shape = self.get_shape()
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j]:
pygame.draw.rect(surface, self.color, (
self.x + j * (BLOCK_SIZE + BLOCK_GAP),
self.y + i * (BLOCK_SIZE + BLOCK_GAP),
BLOCK_SIZE,
BLOCK_SIZE
))
# 定义游戏类
class Game:
def __init__(self):
self.play_area = PLAY_AREA
self.blocks = []
self.current_block = None
self.next_block = Block(
PLAY_WIDTH + BLOCK_SIZE * 2,
BLOCK_SIZE,
random.choice(SHAPES)
)
self.score = 0
self.lines_cleared = 0
self.speed = SPEED
self.state = RUNNING
# 创建新方块
def new_block(self):
self.current_block = self.next_block
self.next_block = Block(
PLAY_WIDTH + BLOCK_SIZE * 2,
BLOCK_SIZE,
random.choice(SHAPES)
)
# 移动方块
def move_block(self, dx, dy):
new_x = self.current_block.x + dx
new_y = self.current_block.y + dy
shape = self.current_block.get_shape()
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j]:
x = new_x + j * (BLOCK_SIZE + BLOCK_GAP)
y = new_y + i * (BLOCK_SIZE + BLOCK_GAP)
if not self.play_area.collidepoint(x, y):
return False
for block in self.blocks:
if block.x == new_x + j * BLOCK_SIZE and block.y == new_y + i * BLOCK_SIZE:
return False
self.current_block.set_position(new_x, new_y)
return True
# 旋转方块
def rotate_block(self):
self.current_block.rotate()
shape = self.current_block.get_shape()
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j]:
x = self.current_block.x + j * (BLOCK_SIZE + BLOCK_GAP)
y = self.current_block.y + i * (BLOCK_SIZE + BLOCK_GAP)
if not self.play_area.collidepoint(x, y):
if self.current_block.x < 0:
self.current_block.set_position(0, self.current_block.y)
elif self.current_block.x + len(shape[i]) * (BLOCK_SIZE + BLOCK_GAP) > PLAY_WIDTH:
self.current_block.set_position(PLAY_WIDTH - len(shape[i]) * (BLOCK_SIZE + BLOCK_GAP), self.current_block.y)
return False
for block in self.blocks:
if block.x == self.current_block.x + j * BLOCK_SIZE and block.y == self.current_block.y + i * BLOCK_SIZE:
if self.current_block.x < block.x:
self.current_block.set_position(block.x - len(shape[i]) * (BLOCK_SIZE + BLOCK_GAP), self.current_block.y)
elif self.current_block.x > block.x:
self.current_block.set_position(block.x + len(shape[i]) * (BLOCK_SIZE + BLOCK_GAP), self.current_block.y)
return False
return True
# 更新游戏状态
def update(self, dt):
if self.state == RUNNING:
if not self.move_block(0, BLOCK_SIZE):
shape = self.current_block.get_shape()
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j]:
x = self.current_block.x + j * (BLOCK_SIZE + BLOCK_GAP)
y = self.current_block.y + i * (BLOCK_SIZE + BLOCK_GAP)
self.blocks.append(Block(x, y, self.current_block.shape))
self.new_block()
self.check_lines()
if not self.move_block(0, 0):
self.state = GAME_OVER
self.speed -= dt
if self.speed <= 0:
self.speed = SPEED
self.move_block(0, BLOCK_SIZE)
# 检查是否有完整的一行
def check_lines(self):
lines = []
for y in range(self.play_area.bottom - BLOCK_SIZE, self.play_area.top - BLOCK_SIZE, -BLOCK_SIZE):
if all(block.y == y for block in self.blocks):
lines.append(y)
if lines:
lines.sort()
for y in lines:
for block in list(self.blocks):
if block.y == y:
self.blocks.remove(block)
for block in list(self.blocks):
if block.y < y:
block.set_position(block.x, block.y + BLOCK_SIZE)
self.score += 10
self.lines_cleared += 1
if self.lines_cleared % 10 == 0:
self.speed -= 50
# 绘制游戏界面
def draw(self, surface):
surface.fill(WHITE)
pygame.draw.rect(surface, BLACK, self.play_area, 5)
for block in self.blocks:
block.draw(surface)
self.current_block.draw(surface)
self.next_block.draw(surface)
score_text = FONT.render(f"Score: {self.score}", True, BLACK)
surface.blit(score_text, (PLAY_WIDTH + BLOCK_SIZE * 2, BLOCK_SIZE * 6))
lines_text = FONT.render(f"Lines: {self.lines_cleared}", True, BLACK)
surface.blit(lines_text, (PLAY_WIDTH + BLOCK_SIZE * 2, BLOCK_SIZE * 8))
if self.state == GAME_OVER:
game_over_text = FONT.render("GAME OVER", True, RED)
surface.blit(game_over_text, (PLAY_WIDTH // 2 - BLOCK_SIZE * 3, PLAY_HEIGHT // 2 - BLOCK_SIZE * 2))
# 创建游戏对象
game = Game()
# 游戏循环
clock = pygame.time.Clock()
done = False
while not done:
dt = clock.tick(FPS)
# 事件处理
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
game.move_block(-BLOCK_SIZE - BLOCK_GAP, 0)
elif event.key == pygame.K_RIGHT:
game.move_block(BLOCK_SIZE + BLOCK_GAP, 0)
elif event.key == pygame.K_DOWN:
game.move_block(0, BLOCK_SIZE + BLOCK_GAP)
elif event.key == pygame.K_UP:
game.rotate_block()
# 更新游戏状态
game.update(dt)
# 绘制游戏界面
game.draw(WINDOW)
pygame.display.flip()
# 退出pygame
pygame.quit()
```
用Python 写一个俄罗斯方块游戏
可以的,你可以使用 Pygame 模块来实现俄罗斯方块游戏。你需要使用 Python 编写游戏逻辑,包括方块的移动、旋转、消除等操作,同时使用 Pygame 来实现游戏界面和音效等功能。你可以在网上找到很多关于如何使用 Pygame 开发游戏的教程和示例代码。
阅读全文
相关推荐
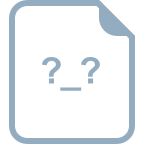
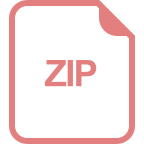

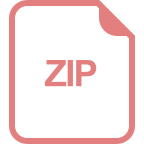
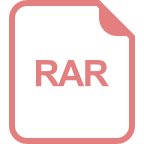
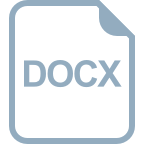
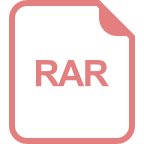