请按以下描述,自定义数据结构,实现一个circular bufferIn computer science, a circular buffer, circular queue, cyclic buffer or ring buffer is a data structure that uses a single,fixed-size buffer as if it were connected end-to-end. This structure lends itself easily to bufering data streams. There were earlycircular buffer implementations in hardware. A circular buffer first starts out empty and has a set length. In the diagram below is a 7-element buffeiAssume that 1 is written in the center of a circular buffer (the exact starting locatiorimportant in a circular buffer)8Then assume that two more elements are added to the circulal2buffers use FIFOlf two elements are removed, the two oldest values inside of the circulal(first in, first out) logic. n the example, 1 8 2 were the first to enter the cremoved,leaving 3 inside of the buffer.If the buffer has 7 elements, then it is completely full6 7 8 5 3 4 5A property of the circular buffer is that when it is full and a subsequent write is performed,overwriting the oldesdata. In the current example, two more elements - A & B - are added and theythe 3 & 475 Alternatively, the routines that manage the buffer could prevent overwriting the data and retur an error or raise an exceptionWhether or not data is overwritten is up to the semantics of the buffer routines or the application using the circular bufer.Finally, if two elements are now removed then what would be retured is not 3 & 4 but 5 8 6 because A & B overwrote the 3 &the 4 yielding the buffer with:
时间: 2024-01-06 20:04:46 浏览: 93
To implement a circular buffer in C++, we can define a class with the following properties:
1. A fixed-size array to hold the buffer elements.
2. Two pointers to keep track of the head and tail of the buffer.
3. A boolean flag to indicate whether the buffer is full or not.
Here is an implementation of a circular buffer class:
```
template<typename T, int N>
class CircularBuffer {
public:
CircularBuffer() : head_(0), tail_(0), full_(false) {}
bool empty() const {
return !full_ && (head_ == tail_);
}
bool full() const {
return full_;
}
int size() const {
int size = N;
if (!full_) {
if (head_ >= tail_)
size = head_ - tail_;
else
size = N + head_ - tail_;
}
return size;
}
void push(const T& value) {
buffer_[head_] = value;
if (full_)
tail_ = (tail_ + 1) % N;
head_ = (head_ + 1) % N;
full_ = (head_ == tail_);
}
T pop() {
if (empty())
throw std::out_of_range("CircularBuffer is empty");
T value = buffer_[tail_];
tail_ = (tail_ + 1) % N;
full_ = false;
return value;
}
private:
T buffer_[N];
int head_;
int tail_;
bool full_;
};
```
To use the circular buffer, we can create an instance of the `CircularBuffer` class with a specific element type and size, like this:
```
CircularBuffer<int, 7> buffer;
```
Then we can push and pop elements from the buffer using the `push` and `pop` methods, like this:
```
buffer.push(1);
buffer.push(8);
buffer.push(2);
int a = buffer.pop();
int b = buffer.pop();
```
The `empty`, `full`, and `size` methods can be used to check the state of the buffer, like this:
```
bool isEmpty = buffer.empty();
bool isFull = buffer.full();
int size = buffer.size();
```
阅读全文
相关推荐
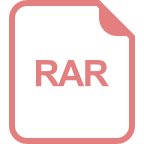
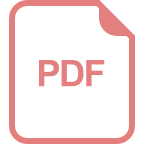

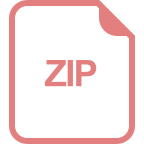
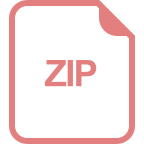
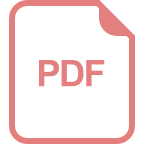
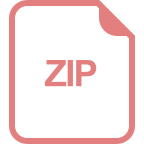
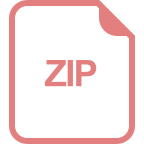
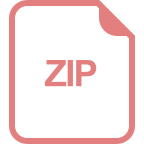
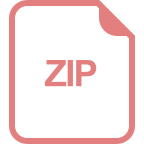
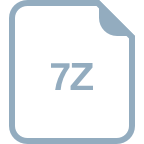
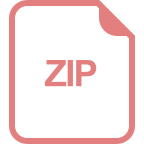
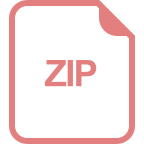
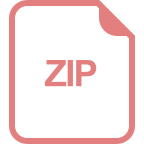
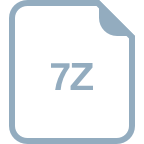
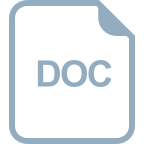
