求一个整数n的所有因子并输出,输出结果在同一行显示,中间用空格分开,n的值从键盘输入用c语言写
时间: 2024-02-24 08:53:10 浏览: 41
好的,以下是C语言的实现代码:
```c
#include <stdio.h>
int main() {
int n;
scanf("%d", &n); // 读入整数n
for (int i = 1; i <= n; i++) { // 枚举所有可能的因子
if (n % i == 0) { // 判断i是否为n的因子
printf("%d ", i); // 输出因子i
}
}
printf("\n"); // 换行
return 0;
}
```
这里我们用到了for循环来枚举所有可能的因子,如果i是n的因子,则输出i。需要注意的是,输出结果中每个因子之间需要用一个空格隔开,最后还需要换行。
相关问题
用c语言求一个整数n的所有因子并输出,输出结果在同一行显示,中间用空格分开,n的值从键盘输入。
```c
#include <stdio.h>
int main() {
int n, i;
printf("请输入一个整数n:");
scanf("%d", &n);
printf("%d的所有因子为:", n);
for (i = 1; i <= n; i++) {
if (n % i == 0) {
printf("%d ", i);
}
}
return 0;
}
```
用c语言从键盘任意输入一个整数n,编程输出n以内的所有素数,并求出八以内所有素数之和
好的,接下来是我的回答:
您好!感谢使用 ChitGPT 与我进行对话,我将为您解答您的问题。
以下是用 C 语言编写输出 n 以内的所有素数以及求出八以内所有素数之和的代码:
```c
#include <stdio.h>
int isPrime(int n) { // 判断是否为素数函数
int i;
if(n <= 1) { // 特判1和0
return 0;
}
for(i = 2; i * i <= n; i++) {
if(n % i == 0) { // 如果能被整除,说明不是素数
return 0;
}
}
return 1; // 是素数
}
int main() {
int n;
int sum = 0; // 存储八以内所有素数之和
printf("请输入一个整数 n:");
scanf("%d", &n); // 输入 n
printf("%d 以内的所有素数为:", n);
for(int i = 2; i <= n; i++) {
if(isPrime(i)) {
printf("%d ", i);
if(i<=8) { // 如果小于等于八,就加上
sum += i;
}
}
}
printf("\n八以内所有素数之和为:%d", sum);
return 0;
}
```
请注意,该代码中的函数用于判断一个给定的整数是否为素数。对于给定的 n 值,该程序利用一个 for 循环结构循环遍历 2 到 n 之间的每个整数。对于每个整数 i,它使用 isPrime 函数检查它是否为素数,如果是素数就输出并将该素数的值加到 sum 变量中(如果它小于等于 8)。最后,程序输出求和结果。
希望我的回答可以帮助您!如果您还有其他问题或需求,请随时向我提出!
相关推荐
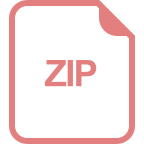
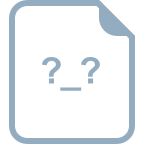
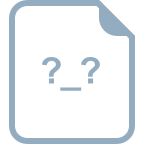












