新闻系统_基于SSM框架新闻系统附带详细教程
时间: 2024-01-11 10:03:29 浏览: 37
这个新闻系统是一个基于SSM框架(Spring+SpringMVC+MyBatis)的Web应用程序,用于管理和发布新闻。它包含了一个完整的用户界面,通过这个界面,用户可以浏览和搜索新闻,以及查看新闻的详细信息。管理员可以登录后台管理系统,添加、编辑和删除新闻,管理新闻分类、标签、用户等信息。下面是详细的教程:
1. 环境准备
首先需要准备好开发环境,包括Java、Tomcat服务器、MySQL数据库和Maven构建工具。Java版本建议使用1.8以上,Tomcat版本建议使用8.0以上,MySQL版本建议使用5.7以上。
2. 创建数据库
在MySQL数据库中创建一个名为“news”的数据库,并在该数据库中创建以下表:
- news_category: 新闻分类表,包含id、name、description三个字段;
- news_tag: 新闻标签表,包含id、name、description三个字段;
- news_user: 新闻用户表,包含id、username、password、email、phone、role、status七个字段;
- news_article: 新闻文章表,包含id、title、content、category_id、tag_ids、create_time、update_time、user_id七个字段。
这些表可以使用以下SQL语句进行创建:
```
CREATE TABLE news_category (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
description VARCHAR(255),
PRIMARY KEY (id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
CREATE TABLE news_tag (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
description VARCHAR(255),
PRIMARY KEY (id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
CREATE TABLE news_user (
id INT(11) NOT NULL AUTO_INCREMENT,
username VARCHAR(50) NOT NULL,
password VARCHAR(50) NOT NULL,
email VARCHAR(50) NOT NULL,
phone VARCHAR(20),
role INT(1) NOT NULL DEFAULT '0',
status INT(1) NOT NULL DEFAULT '0',
PRIMARY KEY (id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
CREATE TABLE news_article (
id INT(11) NOT NULL AUTO_INCREMENT,
title VARCHAR(255) NOT NULL,
content TEXT NOT NULL,
category_id INT(11) NOT NULL,
tag_ids VARCHAR(255),
create_time DATETIME NOT NULL,
update_time DATETIME NOT NULL,
user_id INT(11) NOT NULL,
PRIMARY KEY (id),
KEY category_id (category_id),
KEY user_id (user_id),
CONSTRAINT news_article_ibfk_1 FOREIGN KEY (category_id) REFERENCES news_category (id),
CONSTRAINT news_article_ibfk_2 FOREIGN KEY (user_id) REFERENCES news_user (id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
3. 创建项目
使用Maven创建一个新的Maven项目,并添加SSM框架的相关依赖(spring、spring-webmvc、mybatis、mybatis-spring),并将MySQL的JDBC驱动程序添加到项目中。
4. 配置文件
在src/main/resources目录下创建一个名为“jdbc.properties”的配置文件,其中包含数据库的连接信息:
```
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/news?useUnicode=true&characterEncoding=utf8
jdbc.username=root
jdbc.password=123456
```
在src/main/resources目录下创建一个名为“mybatis-config.xml”的文件,用于配置MyBatis框架的相关信息:
```
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<settings>
<setting name="cacheEnabled" value="true"/>
<setting name="lazyLoadingEnabled" value="true"/>
<setting name="multipleResultSetsEnabled" value="true"/>
<setting name="useColumnLabel" value="true"/>
<setting name="useGeneratedKeys" value="false"/>
<setting name="autoMappingBehavior" value="PARTIAL"/>
<setting name="defaultExecutorType" value="SIMPLE"/>
<setting name="defaultStatementTimeout" value="25"/>
<setting name="safeRowBoundsEnabled" value="false"/>
<setting name="mapUnderscoreToCamelCase" value="false"/>
<setting name="localCacheScope" value="SESSION"/>
<setting name="jdbcTypeForNull" value="OTHER"/>
<setting name="lazyLoadTriggerMethods"
value="equals,clone,hashCode,toString"/>
</settings>
<typeAliases>
<package name="com.example.news.entity"/>
</typeAliases>
<mappers>
<mapper resource="mapper/CategoryMapper.xml"/>
<mapper resource="mapper/TagMapper.xml"/>
<mapper resource="mapper/UserMapper.xml"/>
<mapper resource="mapper/ArticleMapper.xml"/>
</mappers>
</configuration>
```
在src/main/resources目录下创建一个名为“spring-mvc.xml”的Spring MVC配置文件,用于配置Spring MVC框架的相关信息:
```
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd">
<!-- 配置扫描包 -->
<context:component-scan base-package="com.example.news.controller"/>
<!-- 配置视图解析器 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/"/>
<property name="suffix" value=".jsp"/>
</bean>
<!-- 配置文件上传解析器 -->
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="defaultEncoding" value="UTF-8"/>
<property name="maxUploadSize" value="10485760"/> <!-- 10MB -->
</bean>
<!-- 配置静态资源处理器 -->
<mvc:resources mapping="/resources/**" location="/resources/"/>
<!-- 配置拦截器 -->
<mvc:interceptors>
<bean class="com.example.news.interceptor.LoginInterceptor"/>
</mvc:interceptors>
</beans>
```
在src/main/resources目录下创建一个名为“spring-mybatis.xml”的Spring和MyBatis整合的配置文件,用于配置Spring和MyBatis框架的相关信息:
```
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.0.xsd">
<!-- 配置扫描包 -->
<context:component-scan base-package="com.example.news.dao"/>
<!-- 配置数据源 -->
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="${jdbc.driver}"/>
<property name="url" value="${jdbc.url}"/>
<property name="username" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
<property name="initialSize" value="5"/>
<property name="maxActive" value="20"/>
<property name="maxIdle" value="10"/>
<property name="minIdle" value="5"/>
<property name="maxWait" value="30000"/>
</bean>
<!-- 配置事务管理器 -->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
<!-- 配置MyBatis -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="configLocation" value="classpath:mybatis-config.xml"/>
</bean>
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.example.news.dao"/>
</bean>
<!-- 开启事务注解 -->
<tx:annotation-driven transaction-manager="transactionManager"/>
</beans>
```
5. 创建实体类
在com.example.news.entity包下创建实体类Category、Tag、User和Article,分别对应新闻分类、新闻标签、新闻用户和新闻文章。
6. 创建DAO层接口和映射文件
在com.example.news.dao包下创建接口CategoryDao、TagDao、UserDao和ArticleDao,分别对应新闻分类、新闻标签、新闻用户和新闻文章的数据访问层接口。
在src/main/resources/mapper目录下创建CategoryMapper.xml、TagMapper.xml、UserMapper.xml和ArticleMapper.xml四个映射文件,分别对应新闻分类、新闻标签、新闻用户和新闻文章的SQL语句。
7. 创建Service层接口和实现类
在com.example.news.service包下创建接口CategoryService、TagService、UserService和ArticleService,分别对应新闻分类、新闻标签、新闻用户和新闻文章的业务逻辑层接口。
在com.example.news.service.impl包下创建实现类CategoryServiceImpl、TagServiceImpl、UserServiceImpl和ArticleServiceImpl,分别对应新闻分类、新闻标签、新闻用户和新闻文章的业务逻辑层实现类。
8. 创建Controller层
在com.example.news.controller包下创建CategoryController、TagController、UserController和ArticleController四个控制器,分别对应新闻分类、新闻标签、新闻用户和新闻文章的控制器。
9. 创建JSP页面
在src/main/webapp/WEB-INF/views目录下创建category、tag、user和article四个子目录,分别对应新闻分类、新闻标签、新闻用户和新闻文章的视图层。
在这些子目录下创建对应的JSP文件,如category/list.jsp、category/add.jsp等。
10. 编写拦截器
在com.example.news.interceptor包下创建LoginInterceptor拦截器,用于拦截未登录的用户访问后台管理系统。
11. 运行项目
将项目部署到Tomcat服务器上,并访问http://localhost:8080/news访问首页。如果要访问后台管理系统,需要先登录。
以上就是一个基于SSM框架的新闻系统的详细教程。
相关推荐
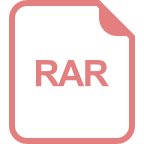
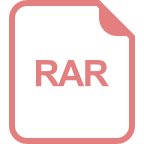
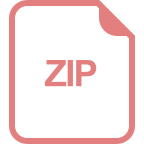
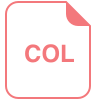
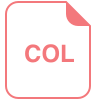
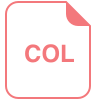
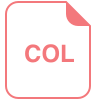
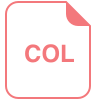









