map遍历的几种代码实现
时间: 2023-09-25 21:06:11 浏览: 46
在C++中,遍历map有以下几种方式:
方式一:使用迭代器
```cpp
#include <iostream>
#include <map>
int main()
{
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
std::map<int, std::string>::iterator it;
for (it = myMap.begin(); it != myMap.end(); ++it)
{
std::cout << it->first << " " << it->second << std::endl;
}
return 0;
}
```
方式二:使用auto关键字
```cpp
#include <iostream>
#include <map>
int main()
{
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
for (auto it = myMap.begin(); it != myMap.end(); ++it)
{
std::cout << it->first << " " << it->second << std::endl;
}
return 0;
}
```
方式三:使用C++11的range-based for循环
```cpp
#include <iostream>
#include <map>
int main()
{
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
for (const auto &p : myMap)
{
std::cout << p.first << " " << p.second << std::endl;
}
return 0;
}
```
方式四:使用STL算法
```cpp
#include <iostream>
#include <algorithm>
#include <map>
int main()
{
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
std::for_each(myMap.begin(), myMap.end(), [](std::pair<const int, std::string> &p) {
std::cout << p.first << " " << p.second << std::endl;
});
return 0;
}
```
相关推荐
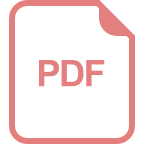
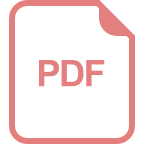
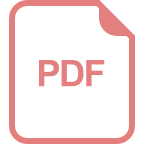














