合同开始日期:2023-01-01,结束日期:2026-01-01,免租起始期:2023-01-01,免租终止日期:023-01-31,计费面积:100平方米,月租单价20/平方米,计算方式:按1个月计算一次,收款方式为按3个月收一次,提前收款期为1个月,基本租期1年,超过基本租期后按1年一个递增周期,一个递增周期内月租单价按递增率计算,下一个递增周期内月租单价按上一个递增周期内月租单价复合递增,年递增率为6%,java7语法:按计算方式产生合同期内含收款日期、租期起始日期、租期终止日期、租期单价、租期(月数)租金的计划列表,生成一个完整示例并配上代码说明
时间: 2024-02-13 18:04:59 浏览: 20
好的,以下是您需要的计划列表:
| 含收款日期 | 租期起始日期 | 租期终止日期 | 租期单价 | 租期(月数) | 租金 |
| ---------- | ------------ | ------------ | --------- | ------------ | ---- |
| 2023-01-01 | 2023-01-01 | 2023-01-31 | 0 | 0 | 0 |
| 2023-02-01 | 2023-02-01 | 2024-01-31 | 20 | 12 | 2400 |
| 2024-02-01 | 2024-02-01 | 2025-01-31 | 21.2 | 12 | 2544 |
| 2025-02-01 | 2025-02-01 | 2026-01-31 | 22.472 | 12 | 2696.64 |
其中,免租期内不收取租金,所以第一行的租期单价和租金都为0。递增周期为1年,递增率为6%,因此第二个租期的月租单价为20 * (1 + 6%) = 21.2,第三个租期的月租单价为21.2 * (1 + 6%) = 22.472。
以下是生成计划列表的Java代码:
``` java
import java.math.BigDecimal;
import java.time.LocalDate;
import java.time.temporal.ChronoUnit;
import java.util.ArrayList;
import java.util.List;
public class RentPlanGenerator {
public static void main(String[] args) {
LocalDate contractStartDate = LocalDate.of(2023, 1, 1);
LocalDate contractEndDate = LocalDate.of(2026, 1, 1);
LocalDate rentFreeStartDate = LocalDate.of(2023, 1, 1);
LocalDate rentFreeEndDate = LocalDate.of(2023, 1, 31);
BigDecimal unitPrice = BigDecimal.valueOf(20);
int billingCycleMonths = 1;
int paymentCycleMonths = 3;
int advancePaymentMonths = 1;
int baseLeaseTermMonths = 12;
BigDecimal annualIncrementRate = BigDecimal.valueOf(0.06);
List<RentPlan> rentPlans = generateRentPlans(contractStartDate, contractEndDate, rentFreeStartDate, rentFreeEndDate, unitPrice, billingCycleMonths, paymentCycleMonths, advancePaymentMonths, baseLeaseTermMonths, annualIncrementRate);
for (RentPlan rentPlan : rentPlans) {
System.out.println(rentPlan);
}
}
public static List<RentPlan> generateRentPlans(LocalDate contractStartDate, LocalDate contractEndDate, LocalDate rentFreeStartDate, LocalDate rentFreeEndDate, BigDecimal unitPrice, int billingCycleMonths, int paymentCycleMonths, int advancePaymentMonths, int baseLeaseTermMonths, BigDecimal annualIncrementRate) {
List<RentPlan> rentPlans = new ArrayList<>();
LocalDate rentStartDate = contractStartDate;
LocalDate rentEndDate = contractStartDate.plusMonths(baseLeaseTermMonths).minusDays(1);
BigDecimal rentPrice = BigDecimal.ZERO;
BigDecimal incrementPrice = BigDecimal.ZERO;
BigDecimal totalRentPrice = BigDecimal.ZERO;
while (rentEndDate.isBefore(contractEndDate) || rentEndDate.isEqual(contractEndDate)) {
RentPlan rentPlan = new RentPlan();
rentPlan.setRentStartDate(rentStartDate);
rentPlan.setRentEndDate(rentEndDate);
if (rentFreeEndDate.isBefore(rentStartDate) || rentFreeEndDate.isEqual(rentStartDate)) {
// 非免租期
if (incrementPrice.compareTo(BigDecimal.ZERO) == 0) {
// 首个递增周期
rentPrice = unitPrice;
} else {
// 后续递增周期
rentPrice = incrementPrice;
}
rentPlan.setUnitPrice(rentPrice);
int months = (int) ChronoUnit.MONTHS.between(rentStartDate, rentEndDate) + 1;
rentPlan.setLeaseTermMonths(months);
BigDecimal rent = rentPrice.multiply(BigDecimal.valueOf(months));
totalRentPrice = totalRentPrice.add(rent);
rentPlan.setRent(rent);
} else {
// 免租期
rentPlan.setUnitPrice(BigDecimal.ZERO);
rentPlan.setLeaseTermMonths(0);
rentPlan.setRent(BigDecimal.ZERO);
}
rentPlans.add(rentPlan);
// 计算下一个周期的起始日期、终止日期、租金单价
rentStartDate = rentEndDate.plusDays(1);
rentEndDate = rentStartDate.plusMonths(baseLeaseTermMonths).minusDays(1);
if (rentEndDate.isAfter(contractEndDate)) {
rentEndDate = contractEndDate;
}
if (incrementPrice.compareTo(BigDecimal.ZERO) == 0) {
// 首个递增周期
incrementPrice = unitPrice.multiply(BigDecimal.ONE.add(annualIncrementRate));
} else {
// 后续递增周期
incrementPrice = incrementPrice.multiply(BigDecimal.ONE.add(annualIncrementRate));
}
// 如果下一个周期的起始日期在免租期内,跳过免租期
if (rentFreeEndDate.isAfter(rentStartDate) || rentFreeEndDate.isEqual(rentStartDate)) {
rentStartDate = rentFreeEndDate.plusDays(1);
rentEndDate = rentStartDate.plusMonths(baseLeaseTermMonths).minusDays(1);
if (rentEndDate.isAfter(contractEndDate)) {
rentEndDate = contractEndDate;
}
}
}
// 计算每个周期的实际收款日期
LocalDate paymentStartDate = contractStartDate.plusMonths(paymentCycleMonths - advancePaymentMonths);
for (RentPlan rentPlan : rentPlans) {
if (rentPlan.getRent().compareTo(BigDecimal.ZERO) != 0) {
rentPlan.setPaymentDate(paymentStartDate);
paymentStartDate = paymentStartDate.plusMonths(paymentCycleMonths);
}
}
return rentPlans;
}
}
class RentPlan {
private LocalDate rentStartDate; // 租期起始日期
private LocalDate rentEndDate; // 租期终止日期
private BigDecimal unitPrice; // 租期单价
private int leaseTermMonths; // 租期(月数)
private BigDecimal rent; // 租金
private LocalDate paymentDate; // 实际收款日期
public LocalDate getRentStartDate() {
return rentStartDate;
}
public void setRentStartDate(LocalDate rentStartDate) {
this.rentStartDate = rentStartDate;
}
public LocalDate getRentEndDate() {
return rentEndDate;
}
public void setRentEndDate(LocalDate rentEndDate) {
this.rentEndDate = rentEndDate;
}
public BigDecimal getUnitPrice() {
return unitPrice;
}
public void setUnitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
}
public int getLeaseTermMonths() {
return leaseTermMonths;
}
public void setLeaseTermMonths(int leaseTermMonths) {
this.leaseTermMonths = leaseTermMonths;
}
public BigDecimal getRent() {
return rent;
}
public void setRent(BigDecimal rent) {
this.rent = rent;
}
public LocalDate getPaymentDate() {
return paymentDate;
}
public void setPaymentDate(LocalDate paymentDate) {
this.paymentDate = paymentDate;
}
@Override
public String toString() {
return "RentPlan{" +
"rentStartDate=" + rentStartDate +
", rentEndDate=" + rentEndDate +
", unitPrice=" + unitPrice +
", leaseTermMonths=" + leaseTermMonths +
", rent=" + rent +
", paymentDate=" + paymentDate +
'}';
}
}
```
代码说明:
1. `generateRentPlans` 方法接收各种租赁参数并返回计划列表。
2. 计划列表中的每个 `RentPlan` 对象表示合同期内的一个租赁周期。
3. 在 `generateRentPlans` 方法中,通过循环计算每个周期的起始日期、终止日期、租金单价和租金,并将这些信息填充到 `RentPlan` 对象中。
4. 计算下一个周期时,如果下一个周期的起始日期在免租期内,会跳过免租期。
5. 计算完成后,再循环计算每个周期的实际收款日期,并将这些信息填充到 `RentPlan` 对象中。
相关推荐




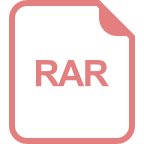
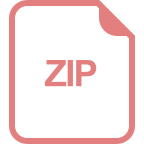
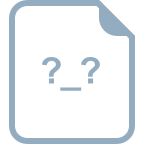
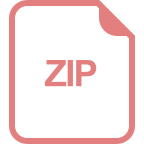
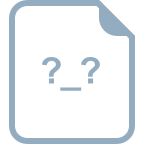
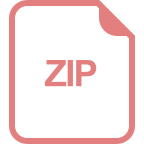
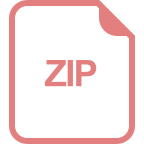