transpose convolution layer作用
时间: 2023-11-04 19:54:59 浏览: 102
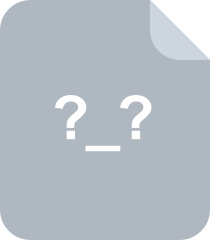
matrix transpose.cpp
Transpose convolution layer(转置卷积层)是一种常用的卷积神经网络(CNN)的层类型,它通常用于图像分割、图像生成等任务中。
在卷积神经网络中,卷积层可以通过卷积核对输入进行卷积操作,从而生成一组特征图。而转置卷积层则是将这个过程反过来,可以将一组特征图通过转置卷积核(也称为反卷积核)进行转置卷积操作,从而将特征图进行上采样,得到更高分辨率的输出特征图。
转置卷积层的作用是扩大特征图的尺寸,从而实现上采样操作,同时也可以学习到一些特定的模式,使得生成的图像更加逼真。转置卷积层通常与卷积层一起使用,构成一个编码器-解码器(Encoder-Decoder)的结构,用于图像生成、图像分割等任务中。
阅读全文
相关推荐
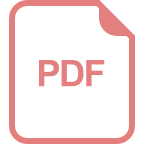
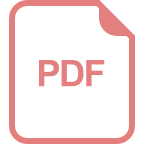



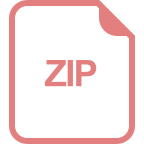
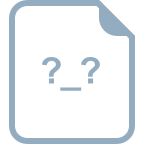
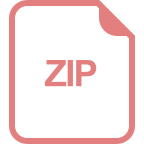
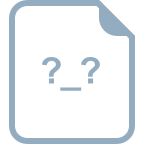
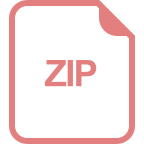
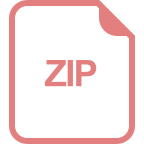