写一个web贪吃蛇小游戏
时间: 2024-01-06 07:04:42 浏览: 107
好的,以下是一个简单的Web贪吃蛇小游戏的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇小游戏</title>
<style>
#game-board {
width: 400px;
height: 400px;
border: 1px solid black;
position: relative;
}
.snake {
width: 20px;
height: 20px;
background-color: green;
position: absolute;
}
.food {
width: 20px;
height: 20px;
background-color: red;
position: absolute;
}
</style>
</head>
<body>
<div id="game-board">
<div class="snake" id="snake"></div>
<div class="food" id="food"></div>
</div>
<script>
// 获取游戏区域和蛇头元素
const gameBoard = document.getElementById('game-board');
const snakeHead = document.getElementById('snake');
// 初始化蛇头位置和方向
let snakeX = 0;
let snakeY = 0;
let snakeDirection = 'right';
// 初始化食物位置
let foodX = 100;
let foodY = 100;
// 监听键盘事件,改变蛇头方向
document.addEventListener('keydown', (event) => {
if (event.key === 'ArrowUp' && snakeDirection !== 'down') {
snakeDirection = 'up';
} else if (event.key === 'ArrowDown' && snakeDirection !== 'up') {
snakeDirection = 'down';
} else if (event.key === 'ArrowLeft' && snakeDirection !== 'right') {
snakeDirection = 'left';
} else if (event.key === 'ArrowRight' && snakeDirection !== 'left') {
snakeDirection = 'right';
}
});
// 更新蛇头位置
function updateSnake() {
if (snakeDirection === 'up') {
snakeY -= 20;
} else if (snakeDirection === 'down') {
snakeY += 20;
} else if (snakeDirection === 'left') {
snakeX -= 20;
} else if (snakeDirection === 'right') {
snakeX += 20;
}
// 检查是否吃到食物
if (snakeX === foodX && snakeY === foodY) {
// 生成新的食物位置
foodX = Math.floor(Math.random() * 20) * 20;
foodY = Math.floor(Math.random() * 20) * 20;
// 创建新的食物元素
const food = document.createElement('div');
food.className = 'food';
food.style.left = foodX + 'px';
food.style.top = foodY + 'px';
gameBoard.appendChild(food);
} else {
// 移除蛇尾元素
gameBoard.removeChild(gameBoard.lastChild);
}
// 创建新的蛇头元素
const snake = document.createElement('div');
snake.className = 'snake';
snake.style.left = snakeX + 'px';
snake.style.top = snakeY + 'px';
gameBoard.appendChild(snake);
}
// 游戏循环
setInterval(() => {
updateSnake();
}, 200);
</script>
</body>
</html>
```
这个示例使用HTML、CSS和JavaScript实现了一个简单的贪吃蛇小游戏。游戏区域以及蛇头和食物元素都通过HTML的`<div>`标签创建,并使用CSS进行样式设置。JavaScript部分处理游戏逻辑,包括监听键盘事件、更新蛇头位置、检测是否吃到食物等。游戏循环使用`setInterval`函数定时调用`updateSnake`函数来更新蛇的位置。您可以根据需要进行扩展和修改,添加更多的游戏功能和交互效果。
阅读全文
相关推荐
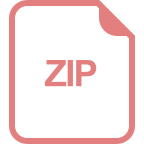
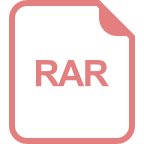
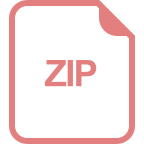
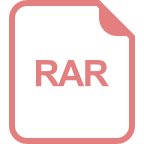
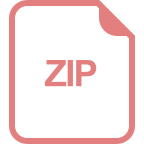
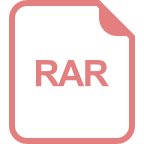
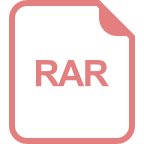
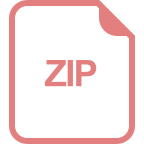
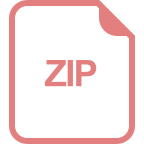
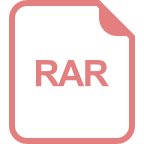
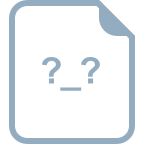
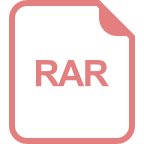
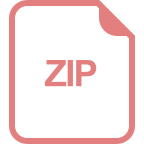
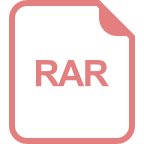
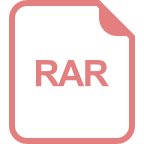
