xamarin在mainviewmodel中跳转到其他页面
时间: 2024-04-10 19:34:37 浏览: 97
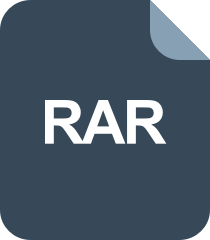
页面跳转
要在 Xamarin 的 MainViewModel 中跳转到其他页面,你可以使用依赖注入来获取当前的导航服务,然后使用该导航服务来执行页面导航操作。
首先,在你的应用程序的启动代码中注册导航服务:
```csharp
DependencyService.Register<INavigationService, NavigationService>();
```
然后,创建一个名为 `INavigationService` 的接口,定义页面导航的方法签名:
```csharp
public interface INavigationService
{
Task NavigateToAsync<TViewModel>() where TViewModel : ViewModelBase;
Task NavigateToAsync<TViewModel>(object parameter) where TViewModel : ViewModelBase;
Task GoBackAsync();
}
```
接下来,创建一个名为 `NavigationService` 的类,实现 `INavigationService` 接口,并在其中使用 `Application.Current.MainPage.Navigation` 获取当前页面的导航对象:
```csharp
public class NavigationService : INavigationService
{
public async Task NavigateToAsync<TViewModel>() where TViewModel : ViewModelBase
{
var page = CreatePage(typeof(TViewModel));
var viewModel = Activator.CreateInstance(typeof(TViewModel));
((Page)page).BindingContext = viewModel;
await Application.Current.MainPage.Navigation.PushAsync((Page)page);
}
public async Task NavigateToAsync<TViewModel>(object parameter) where TViewModel : ViewModelBase
{
var page = CreatePage(typeof(TViewModel));
var viewModel = Activator.CreateInstance(typeof(TViewModel), parameter);
((Page)page).BindingContext = viewModel;
await Application.Current.MainPage.Navigation.PushAsync((Page)page);
}
public async Task GoBackAsync()
{
await Application.Current.MainPage.Navigation.PopAsync();
}
private Page CreatePage(Type viewModelType)
{
var pageType = GetPageTypeForViewModel(viewModelType);
if (pageType == null)
throw new Exception($"Cannot locate page type for {viewModelType}");
return Activator.CreateInstance(pageType) as Page;
}
private Type GetPageTypeForViewModel(Type viewModelType)
{
var viewName = viewModelType.FullName.Replace("ViewModel", "Page");
var viewType = Assembly.GetExecutingAssembly().GetType(viewName);
return viewType;
}
}
```
现在,在你的 MainViewModel 中,通过使用 `DependencyService.Get<INavigationService>()` 来获取 `INavigationService` 的实例,并调用导航方法来跳转到其他页面:
```csharp
public class MainViewModel : ViewModelBase
{
private readonly INavigationService _navigationService;
public MainViewModel()
{
_navigationService = DependencyService.Get<INavigationService>();
}
private async Task NavigateToOtherPage()
{
await _navigationService.NavigateToAsync<OtherViewModel>();
}
}
```
通过这种方式,你可以在 MainViewModel 中使用导航服务来跳转到其他页面。确保你已经正确引用了 `Xamarin.Forms` 命名空间、注册了导航服务,并在适当的地方调用导航方法,以实现页面间的导航。
阅读全文
相关推荐
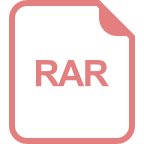
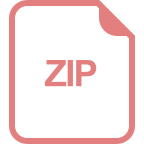




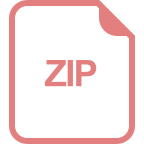
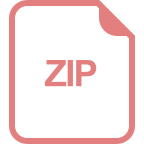
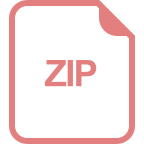




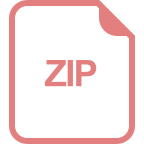


