如何避免这种`AttributeError`?
时间: 2024-09-15 08:02:54 浏览: 32
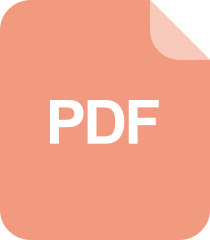
多线程爬虫出现报错AttributeError: ‘NoneType’ object has no attribute ‘xpath’
要避免`AttributeError`,特别是当涉及到对象属性访问时,可以采取以下几种策略:
1. **确保对象已正确初始化**:在使用之前,确认你已经正确地创建并初始化了所需的对象,比如`Screen`或`Turtle`对象。
```python
import turtle
# 确保已经创建了Screen实例
screen = turtle.Screen()
```
2. **检查API文档**:查阅库的官方文档,确认你需要使用的对象是否真的有你想要的属性。比如对于`turtle.Screen`,确认其是否有`width`、`height`等属性。
3. **使用`hasattr()`函数**:你可以使用`hasattr()`函数来检查对象是否具有特定属性,如果返回`True`则可以安全地访问。
```python
if hasattr(screen, 'width'):
width = screen.width
else:
print("Attribute not found")
```
4. **异常处理**:使用`try-except`结构捕获并处理可能发生的`AttributeError`。
```python
try:
width = screen.width
except AttributeError as e:
print(f"An error occurred: {str(e)}")
```
5. **更新库版本**:有时候,旧版的库可能缺少新的属性,确保你正在使用的库版本是最新的。
通过以上步骤,你可以减少遇到`AttributeError`的概率,并更有效地调试代码。
阅读全文
相关推荐
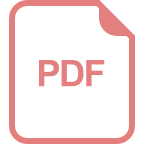
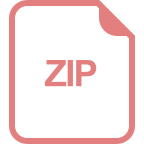















