C++实现词法分析程序,并将代码和运行结果截图上传: 输入:从文件输入一段代码(多行) 输出:代码中各个单次的类别,如 输入:int i=3; 输出: (关键字,int) (标识符,i) (运算符,=) (数字,3) (分隔符,;)
时间: 2024-09-24 20:18:09 浏览: 21
在C++中实现词法分析器通常会采用自顶向下、逐字符扫描的方式,利用标准库中的`fstream`读取文件,然后遍历输入流,通过模式匹配识别出各种词法单元(tokens)。这里提供一个简单的基于正则表达式的基础示例,但请注意这只是一个基础版本,实际生产环境中的词法分析器可能会更复杂,使用词典或者解析表等技术。
```cpp
#include <iostream>
#include <fstream>
#include <regex>
#include <string>
// 定义词法单元类别
enum Token {
KEYWORD,
IDENTIFIER,
OPERATOR,
NUMBER,
SEPARATOR
};
// 词法单元结构体
struct Lexeme {
std::string value;
Token type;
};
// 词法分析函数
Lexeme lexemeFromInput(const std::string& input) {
std::smatch match;
Lexeme lexeme;
// 使用正则表达式匹配
std::regex keywords{"[a-z_]+", std::regex::icase};
std::regex identifier{"[a-zA-Z_][a-zA-Z0-9_]*"};
std::regex operator_regex{"[\\+\\-*/%=<>!?]", std::regex::icase};
std::regex number{"[-+]?\\d+(\\.\\d+)?"};
if (std::regex_search(input, match, keywords)) {
lexeme.type = KEYWORD;
lexeme.value = match.str();
} else if (std::regex_search(input, match, identifier)) {
lexeme.type = IDENTIFIER;
lexeme.value = match.str();
} else if (std::regex_search(input, match, operator_regex)) {
lexeme.type = OPERATOR;
lexeme.value = match.str();
} else if (std::regex_search(input, match, number)) {
lexeme.type = NUMBER;
lexeme.value = match.str();
} else if (input == ";") {
lexeme.type = SEPARATOR;
lexeme.value = input;
} else {
// 如果无法匹配,可以添加错误处理逻辑
throw std::runtime_error("Invalid input");
}
return lexeme;
}
int main() {
std::ifstream inputFile("your_code_file.txt"); // 替换为你要分析的实际文件路径
if (!inputFile) {
std::cerr << "Error opening file" << std::endl;
return 1;
}
std::string line;
while (std::getline(inputFile, line)) {
for (const auto &char : line) {
Lexeme lexeme = lexemeFromInput(std::string(1, char));
std::cout << "(" << lexeme.type << ", " << lexeme.value << ")" << std::endl;
}
}
inputFile.close();
return 0;
}
```
这个代码片段会在每行中逐个字符地进行词法分析并打印出来。为了获取完整的运行结果,你需要替换`main`函数中的`your_code_file.txt`为实际的源代码文件路径,并确保代码能够正常编译和运行。
注意,由于文本格式限制,我无法在这里显示代码执行的结果截图。你可以尝试自己编写代码并在本地环境中运行它来观察输出。
相关推荐
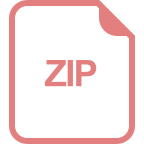
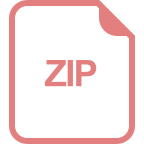
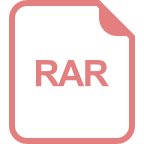














